用c++写将一个首结点指针为a的单链表A分解成两个单链表A和B,其首结点指针分别为a和b,使得链表A中含有原链表A中序号为奇数的元素,而链表B中含有原链表A中序号为偶数的元素,且保持原来的相对顺序。
时间: 2024-10-22 11:27:20 浏览: 28
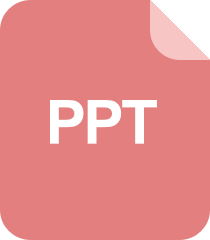
链表结点的C++描述-单链表的实现-简单操作
在C++中,你可以通过遍历链表并根据节点的索引(这里可以视为中序编号,因为对于单链表,中序遍历会得到自然排序的序列)来实现这个操作。以下是步骤:
1. 定义两个空的链表`listA`和`listB`作为新链表的头指针。
2. 使用迭代或递归的方式遍历原链表`A`。对于每个节点,检查它的索引是否为奇数或偶数。
- 如果索引是奇数(`node->index % 2 == 0`),添加到`listA`。
- 否则,添加到`listB`。
3. 在遍历过程中,需要记住当前节点的位置,以便后续找到其相邻的偶数节点(如果有的话)。这通常通过一个临时变量或链表辅助结构来完成。
4. 遍历完成后,分别返回`listA`和`listB`的头指针`a`和`b`。
以下是一个简单的伪代码示例:
```cpp
struct ListNode {
int val;
ListNode* next;
int index; // 添加索引信息
};
ListNode* splitList(ListNode* a) {
ListNode* listA = nullptr, *listB = nullptr;
ListNode* current = a;
ListNode* prev = nullptr;
while (current != nullptr) {
if ((prev ? current->index - prev->index : 0) % 2 == 0) { // 奇数节点
if (!listA) {
listA = current;
} else {
listA->next = current;
listA = listA->next;
}
} else { // 偶数节点
if (!listB) {
listB = current;
} else {
listB->next = current;
listB = listB->next;
}
}
prev = current;
current = current->next;
}
return listA;
}
```
阅读全文
相关推荐
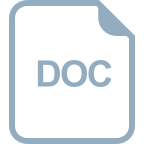
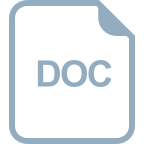
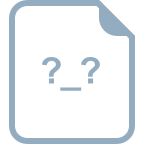











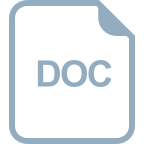
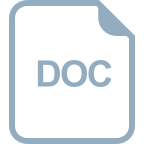
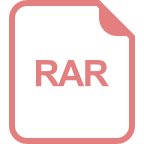