设计一个c++算法,将一个带头结点的单链表A分解为两个具有相同结构的链表B和C,其中B表的结点为A表中价格值小于30的结点,而C表的结点为A表中价格值大于等于30的结点。单链表A中存储的是所有的图书信息。 数据使用数据book.txt。
时间: 2024-09-30 10:08:53 浏览: 41
设计这样的C++算法,我们可以按照以下步骤操作:
首先,我们需要创建链表节点类BookNode,并定义包含价格属性price和指向下一个节点的指针next。然后,我们定义两个链表类ListB和ListC,它们都有各自的头节点。
```cpp
#include <fstream>
#include <iostream>
class BookNode {
public:
int price;
BookNode* next;
// 构造函数、获取和设置价格等其他成员函数...
};
class ListB : public BookNode {
public:
ListB* head;
};
class ListC : public BookNode {
public:
ListC* head;
};
// 函数:读取数据文件并构建BookNode链表
ListB* buildListFromFile(const std::string& filename) {
// 实现从file.txt读取数据,创建BookNode实例并链接到链表中
}
// 函数:分解链表
void splitList(ListB*& listB, ListC*& listC, const ListB* aList) {
if (aList == nullptr) return;
// 将链表分为两部分
BookNode* current = aList->head;
while (current != nullptr) {
if (current->price < 30) {
// 如果价格小于30,将节点添加到listB
// listB->head = current; // 由于链表的头部操作可能需要考虑插入位置,这里暂省略细节
current = current->next;
} else {
// 否则添加到listC
// listC->head = current; // 同上,省略细节
current = current->next;
}
}
}
int main() {
ListB bList;
ListC cList;
ListB* aList = buildListFromFile("data/book.txt");
splitList(bList.head, cList.head, aList);
// 分别处理bList和cList...
// 删除链表头部多余元素,如果需要的话...
// 输出结果...
delete aList;
return 0;
}
```
阅读全文
相关推荐
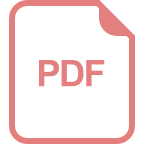
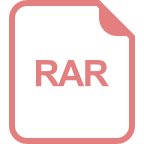
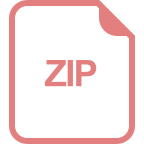













