C++ 设计一个算法,求一个不带头结点的单链表中的结点个数。
时间: 2024-09-27 21:06:43 浏览: 21
在C++中,你可以通过遍历链表的方式来计算其节点个数。这里是一个简单的算法:
```cpp
#include <iostream>
struct Node {
int data; // 节点数据
Node* next; // 指向下一个节点的指针
};
// 函数返回链表的长度
int countNodes(Node* head) {
if (head == nullptr) { // 如果链表为空,则节点个数为0
return 0;
}
int count = 1; // 初始化计数器为1,因为我们已经有一个头节点
Node* current = head->next; // 从第二个节点开始遍历
while (current != nullptr) { // 遍历直到链表结束
count++; // 对每个非空节点增加计数
current = current->next; // 移动到下一个节点
}
return count; // 返回节点总数
}
int main() {
// 创建一个示例链表并调用函数
Node* list = new Node();
list->data = 1;
list->next = new Node(); // 假设链表还有其他节点...
std::cout << "链表节点个数: " << countNodes(list) << std::endl;
// 清理内存
delete list;
return 0;
}
```
在这个算法中,我们首先检查头节点是否为空,如果为空则直接返回0。然后从第二个节点开始遍历整个链表,并在每次迭代中将计数加一,直到遍历完所有节点。
相关推荐
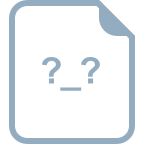
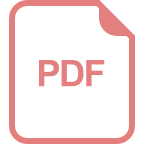
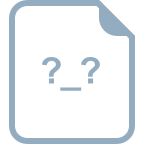














