Cshap Sobel算子算法
时间: 2024-06-12 13:05:32 浏览: 147
Sobel算子是一种图像边缘检测算法,用于检测图像中的边缘。它可以通过对像素点周围的像素点进行加权平均来计算像素点的梯度值,从而确定像素点是否为边缘点。
Sobel算子的计算步骤如下:
1. 将原始图像转换为灰度图像。
2. 对灰度图像进行高斯滤波,以减少噪声。
3. 对每个像素点进行Sobel运算,计算其梯度值。
4. 对梯度值进行二值化,将梯度值大于一个阈值的像素点标记为边缘点,否则标记为非边缘点。
Sobel算子的运算过程中,需要对每个像素点周围的像素点进行加权平均。一般来说,Sobel算子采用3x3的卷积核进行运算,卷积核的权值如下:
```
-1 0 1
-2 0 2
-1 0 1
```
对于每个像素点,Sobel算子将其周围的像素点与卷积核进行卷积运算,得到x方向和y方向的梯度值,分别表示像素点在水平方向和垂直方向的变化程度。最后,将x方向和y方向梯度值的平方和取平方根,得到像素点的总梯度值。
Sobel算子的优点是简单易懂、计算速度快,适合用于实时图像处理等场景。缺点是对噪声敏感,可能会将噪声识别为边缘。
相关问题
Cshap LZW编码算法
LZW编码算法是一种无损压缩算法,它可以将一串字符序列压缩成更短的序列,同时保证压缩前后的数据完全一致。
C#实现LZW算法的步骤如下:
1.建立字典:将所有可能的字符放入一个字典中,初始化时字典包含所有的ASCII字符和一些特殊字符。
2.压缩:从输入字符串中读取字符序列,并将其与字典中的字符进行匹配,每次匹配到一个字符就将其添加到当前匹配序列中,直到匹配不到字符为止。将当前匹配序列的编码输出,并将匹配序列加入字典中。
3.解压:从压缩后的编码序列中读取编码,并在字典中查找对应的字符串。将字符串输出,并将前缀加入字典中。
C#代码实现如下:
//LZW压缩算法
public static List<int> Compress(string input)
{
Dictionary<string, int> dictionary = new Dictionary<string, int>();
for (int i = 0; i < 256; i++)
{
dictionary.Add(((char)i).ToString(), i);
}
string prefix = "";
List<int> output = new List<int>();
foreach (char c in input)
{
string combined = prefix + c;
if (dictionary.ContainsKey(combined))
{
prefix = combined;
}
else
{
output.Add(dictionary[prefix]);
dictionary.Add(combined, dictionary.Count);
prefix = c.ToString();
}
}
output.Add(dictionary[prefix]);
return output;
}
//LZW解压算法
public static string Decompress(List<int> input)
{
Dictionary<int, string> dictionary = new Dictionary<int, string>();
for (int i = 0; i < 256; i++)
{
dictionary.Add(i, ((char)i).ToString());
}
string prefix = dictionary[input[0]];
string output = prefix;
for (int i = 1; i < input.Count; i++)
{
string entry = "";
if (dictionary.ContainsKey(input[i]))
{
entry = dictionary[input[i]];
}
else if (input[i] == dictionary.Count)
{
entry = prefix + prefix[0];
}
output += entry;
dictionary.Add(dictionary.Count, prefix + entry[0]);
prefix = entry;
}
return output;
}
Cshap 烙饼排序算法
烙饼排序(Pancake Sorting)是一种简单的排序算法,其基本思路是通过翻转烙饼来将其排序。以下是C#实现的烙饼排序算法:
```
using System;
public class PancakeSorting
{
public static void Sort(int[] arr)
{
for (int i = arr.Length - 1; i > 0; i--)
{
int maxIndex = FindMaxIndex(arr, i);
if (maxIndex != i)
{
Flip(arr, maxIndex);
Flip(arr, i);
}
}
}
private static int FindMaxIndex(int[] arr, int end)
{
int maxIndex = 0;
for (int i = 1; i <= end; i++)
{
if (arr[i] > arr[maxIndex])
{
maxIndex = i;
}
}
return maxIndex;
}
private static void Flip(int[] arr, int end)
{
int start = 0;
while (start < end)
{
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
}
```
在这个实现中,我们首先找到未排序部分中的最大元素,然后将其翻转到数组的顶部。然后,我们将整个未排序部分翻转,将最大元素翻转到数组的底部。通过这种方式,我们逐步将数组排序。
以下是一个使用示例:
```
int[] arr = { 3, 1, 6, 2, 8, 4 };
PancakeSorting.Sort(arr);
Console.WriteLine(string.Join(", ", arr)); // 输出:1, 2, 3, 4, 6, 8
```
阅读全文
相关推荐
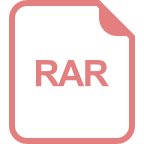
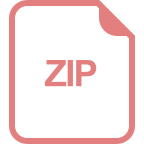
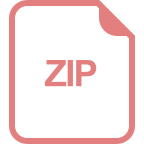













