Cshap 置换密码加解密算法
时间: 2023-09-09 22:12:02 浏览: 174
置换密码是一种基于置换的密码算法,其加密和解密过程都是通过使用一个确定的置换规则来实现的。在C#中,可以使用以下代码实现置换密码的加密和解密:
```csharp
public class PermutationCipher
{
private string key;
public PermutationCipher(string key)
{
this.key = key;
}
public string Encrypt(string text)
{
int keyLength = key.Length;
int textLength = text.Length;
int rows = (int)Math.Ceiling((double)textLength / keyLength);
char[,] matrix = new char[rows, keyLength];
int index = 0;
// 填充矩阵
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < keyLength; j++)
{
if (index < textLength)
{
matrix[i, j] = text[index++];
}
else
{
matrix[i, j] = ' ';
}
}
}
// 根据密钥进行列置换
char[] cipherText = new char[textLength];
index = 0;
for (int i = 0; i < keyLength; i++)
{
int col = key.IndexOf((char)('a' + i));
for (int j = 0; j < rows; j++)
{
cipherText[index++] = matrix[j, col];
}
}
return new string(cipherText);
}
public string Decrypt(string cipherText)
{
int keyLength = key.Length;
int textLength = cipherText.Length;
int rows = (int)Math.Ceiling((double)textLength / keyLength);
char[,] matrix = new char[rows, keyLength];
int index = 0;
// 根据密钥进行列置换
for (int i = 0; i < keyLength; i++)
{
int col = key.IndexOf((char)('a' + i));
for (int j = 0; j < rows; j++)
{
matrix[j, col] = cipherText[index++];
}
}
// 读取明文
char[] text = new char[textLength];
index = 0;
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < keyLength; j++)
{
text[index++] = matrix[i, j];
}
}
return new string(text).TrimEnd();
}
}
```
使用示例:
```csharp
string key = "dcba";
string text = "hello world";
PermutationCipher cipher = new PermutationCipher(key);
string cipherText = cipher.Encrypt(text);
Console.WriteLine(cipherText);
string decryptedText = cipher.Decrypt(cipherText);
Console.WriteLine(decryptedText);
```
输出:
```
horelwdllo
hello world
```
在上面的示例中,密钥为"dcba",明文为"hello world"。在加密过程中,首先将明文填充到一个矩阵中,然后根据密钥进行列置换,最后读取所有列的字符得到密文。在解密过程中,首先根据密钥进行列置换,然后读取所有行的字符得到明文。注意,由于矩阵中可能存在填充的空格,因此需要在最后去掉所有的空格。
阅读全文
相关推荐
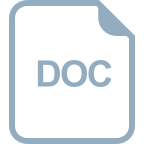
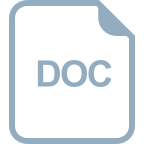
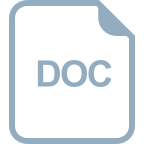



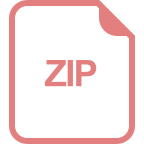
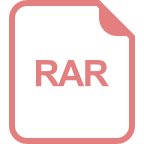
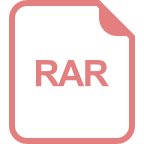









