用python把热力图转化为数据吗?
时间: 2023-10-06 07:04:31 浏览: 131
是的,可以使用Python将热力图转换为数据。一种常用的方法是使用Python的图像处理库,如OpenCV或Pillow来读取和处理热力图。然后,使用NumPy等数学库来将图像转换为数据数组。具体步骤如下:
1. 使用OpenCV或Pillow读取热力图,将其转换为NumPy数组。
```python
import cv2
import numpy as np
img = cv2.imread('heatmap.png')
data = np.asarray(img)
```
2. 将RGB数组转换为灰度数组。
```python
gray = cv2.cvtColor(data, cv2.COLOR_RGB2GRAY)
```
3. 对灰度数组进行阈值处理,将其转换为二进制数组。
```python
ret, binary = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
```
4. 对二进制数组进行形态学处理,以去除噪声并提取出热力图中的点。
```python
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
morphed = cv2.morphologyEx(binary, cv2.MORPH_OPEN, kernel)
points = np.transpose(np.nonzero(morphed))
```
现在,`points`数组包含了热力图中所有的点的坐标。你可以将其保存为CSV文件或使用其他方式进行处理。
相关问题
用python把热力图转化为数据保存在excel表格中
要将热力图转换为数据并将其保存到Excel表格中,可以使用Python的Pandas库。具体步骤如下:
1. 使用Pillow库读取热力图,并将其转换为NumPy数组。
```python
from PIL import Image
import numpy as np
img = Image.open('heatmap.png')
data = np.asarray(img)
```
2. 将RGB数组转换为灰度数组。
```python
gray = np.mean(data, axis=2)
```
3. 对灰度数组进行阈值处理,将其转换为二进制数组。
```python
threshold = 127
binary = np.where(gray > threshold, 1, 0)
```
4. 对二进制数组进行形态学处理,以去除噪声并提取出热力图中的点。
```python
from scipy import ndimage
kernel = np.ones((3, 3), np.uint8)
morphed = ndimage.binary_opening(binary, structure=kernel)
points = np.transpose(np.nonzero(morphed))
```
5. 使用Pandas创建DataFrame,并将点的坐标保存到Excel表格中。
```python
import pandas as pd
df = pd.DataFrame(points, columns=['x', 'y'])
df.to_excel('heatmap_points.xlsx', index=False)
```
现在,你已经将热力图中的点保存到了Excel表格中。你可以使用Excel或其他工具对这些数据进行进一步的分析和可视化。
用python把热力图转化为数据保存在excel表格中,需要完整地实现代码
好的,以下是完整的Python代码,可以将热力图转换为数据并将其保存到Excel表格中:
```python
from PIL import Image
import numpy as np
from scipy import ndimage
import pandas as pd
# 读取热力图并转换为NumPy数组
img = Image.open('heatmap.png')
data = np.asarray(img)
# 将RGB数组转换为灰度数组
gray = np.mean(data, axis=2)
# 对灰度数组进行阈值处理,将其转换为二进制数组
threshold = 127
binary = np.where(gray > threshold, 1, 0)
# 对二进制数组进行形态学处理,以去除噪声并提取出热力图中的点
kernel = np.ones((3, 3), np.uint8)
morphed = ndimage.binary_opening(binary, structure=kernel)
points = np.transpose(np.nonzero(morphed))
# 使用Pandas创建DataFrame,并将点的坐标保存到Excel表格中
df = pd.DataFrame(points, columns=['x', 'y'])
df.to_excel('heatmap_points.xlsx', index=False)
```
请确保将代码中的文件路径替换为你自己的热力图文件路径。此代码将热力图中的点保存为Excel表格中的两列数据,分别表示x和y坐标。你可以根据需要自行修改代码以保存其他信息。
阅读全文
相关推荐
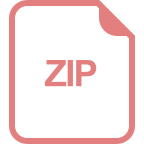




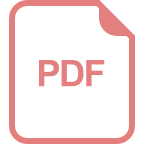
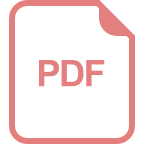
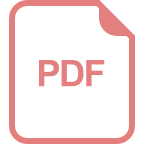
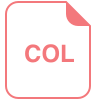





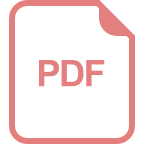