c++反转链表pat
时间: 2023-06-28 12:11:17 浏览: 130
以下是使用C++实现反转链表的代码,可以用于PAT等面试/编程练习。
```c++
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
ListNode* reverseList(ListNode* head) {
ListNode* prev = NULL;
ListNode* curr = head;
while (curr != NULL) {
ListNode* next = curr->next;
curr->next = prev;
prev = curr;
curr = next;
}
return prev;
}
int main() {
// 构造链表 1->2->3->4->5
ListNode* head = new ListNode(1);
head->next = new ListNode(2);
head->next->next = new ListNode(3);
head->next->next->next = new ListNode(4);
head->next->next->next->next = new ListNode(5);
// 反转链表
ListNode* newHead = reverseList(head);
// 输出反转后的链表
while (newHead != NULL) {
cout << newHead->val << ' ';
newHead = newHead->next;
}
cout << endl;
return 0;
}
```
输出结果为:
```
5 4 3 2 1
```
其中,`reverseList` 函数实现了链表的反转,使用了三个指针,分别指向当前节点、前一个节点和后一个节点。在循环中不断更新这三个指针,直到遍历完整个链表。
在 `main` 函数中,我们构造了一个链表,并调用 `reverseList` 函数将其反转,然后输出反转后的链表。
阅读全文
相关推荐
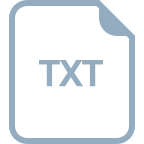
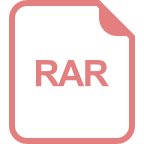
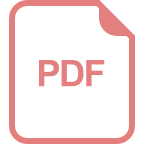
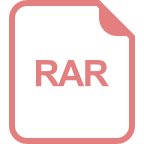
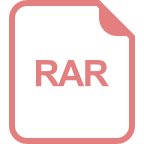
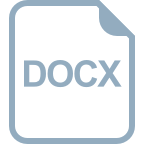
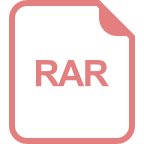
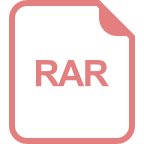
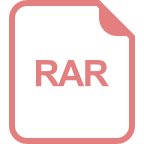
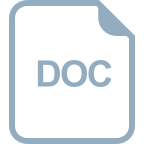
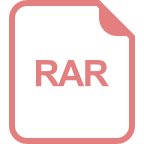