用乌鸦搜索算法优化随机森林模型代码
时间: 2023-06-17 18:02:19 浏览: 67
乌鸦搜索算法(Crow Search Algorithm, CSA)是一种基于群体智能的优化算法。其主要模拟了乌鸦群体中的协作行为,通过群体中个体之间的信息交流和协作来寻找最优解。下面给出一个用乌鸦搜索算法来优化随机森林模型的代码示例。
首先需要导入必要的库和数据集:
```python
import numpy as np
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
from sklearn.model_selection import train_test_split
# 导入数据集
data = pd.read_csv('data.csv')
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
```
接下来定义用于优化的目标函数,即随机森林模型的准确率:
```python
# 定义目标函数
def fitness_function(params):
n_estimators = int(params[0])
max_depth = int(params[1])
max_features = params[2]
criterion = params[3]
# 训练随机森林模型
clf = RandomForestClassifier(n_estimators=n_estimators, max_depth=max_depth,
max_features=max_features, criterion=criterion, random_state=42)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
clf.fit(X_train, y_train)
# 计算准确率作为目标函数值
y_pred = clf.predict(X_test)
return accuracy_score(y_test, y_pred)
```
然后定义乌鸦搜索算法的相关参数:
```python
# 定义乌鸦搜索算法的参数
n_crows = 10 # 种群大小
n_iter = 100 # 迭代次数
pa = 0.25 # 父母选择概率
pc = 0.8 # 交叉概率
pm = 0.1 # 变异概率
lb = [50, 1, 'sqrt', 'gini'] # 搜索空间下界
ub = [100, 10, 'log2', 'entropy'] # 搜索空间上界
```
其中,`n_crows`为种群大小,`n_iter`为迭代次数,`pa`为父母选择概率,`pc`为交叉概率,`pm`为变异概率,`lb`和`ub`分别为搜索空间下界和上界。
接下来定义乌鸦搜索算法的核心代码:
```python
# 初始化种群
population = np.random.uniform(low=lb, high=ub, size=(n_crows, len(lb)))
# 迭代搜索
for i in range(n_iter):
# 计算适应度值
fitness = np.array([fitness_function(params) for params in population])
# 父母选择
sorted_idx = np.argsort(fitness)[::-1]
selected_idx = sorted_idx[:int(pa*n_crows)]
parents = population[selected_idx]
# 交叉
children = np.zeros_like(parents)
for j in range(int(pc*len(parents))):
p1, p2 = np.random.choice(parents.shape[0], size=2, replace=False)
alpha = np.random.uniform(low=-0.5, high=1.5, size=parents.shape[1])
children[j] = parents[p1] + alpha*(parents[p2]-parents[p1])
# 变异
for j in range(int(pm*len(children))):
mutant = np.random.randint(len(parents))
alpha = np.random.uniform(low=-0.5, high=1.5, size=parents.shape[1])
children[mutant] = children[mutant] + alpha*(ub-lb)
# 合并种群
population = np.vstack((population, children))
# 修剪种群
fitness = np.array([fitness_function(params) for params in population])
sorted_idx = np.argsort(fitness)[::-1]
population = population[sorted_idx[:n_crows]]
```
首先初始化种群,并计算每个个体的适应度值。然后进行父母选择,选出适应度值较高的个体作为父母。接着进行交叉操作,生成新的个体。最后进行变异操作,并将新个体与原有个体合并。修剪种群后,进行下一轮迭代。
最后输出最优解:
```python
# 输出最优解
fitness = np.array([fitness_function(params) for params in population])
best_idx = np.argmax(fitness)
best_params = population[best_idx]
best_fitness = fitness[best_idx]
print('Best params:', best_params)
print('Best fitness:', best_fitness)
```
完整代码如下所示:
```python
import numpy as np
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
from sklearn.model_selection import train_test_split
# 导入数据集
data = pd.read_csv('data.csv')
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
# 定义目标函数
def fitness_function(params):
n_estimators = int(params[0])
max_depth = int(params[1])
max_features = params[2]
criterion = params[3]
# 训练随机森林模型
clf = RandomForestClassifier(n_estimators=n_estimators, max_depth=max_depth,
max_features=max_features, criterion=criterion, random_state=42)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
clf.fit(X_train, y_train)
# 计算准确率作为目标函数值
y_pred = clf.predict(X_test)
return accuracy_score(y_test, y_pred)
# 定义乌鸦搜索算法的参数
n_crows = 10 # 种群大小
n_iter = 100 # 迭代次数
pa = 0.25 # 父母选择概率
pc = 0.8 # 交叉概率
pm = 0.1 # 变异概率
lb = [50, 1, 'sqrt', 'gini'] # 搜索空间下界
ub = [100, 10, 'log2', 'entropy'] # 搜索空间上界
# 初始化种群
population = np.random.uniform(low=lb, high=ub, size=(n_crows, len(lb)))
# 迭代搜索
for i in range(n_iter):
# 计算适应度值
fitness = np.array([fitness_function(params) for params in population])
# 父母选择
sorted_idx = np.argsort(fitness)[::-1]
selected_idx = sorted_idx[:int(pa*n_crows)]
parents = population[selected_idx]
# 交叉
children = np.zeros_like(parents)
for j in range(int(pc*len(parents))):
p1, p2 = np.random.choice(parents.shape[0], size=2, replace=False)
alpha = np.random.uniform(low=-0.5, high=1.5, size=parents.shape[1])
children[j] = parents[p1] + alpha*(parents[p2]-parents[p1])
# 变异
for j in range(int(pm*len(children))):
mutant = np.random.randint(len(parents))
alpha = np.random.uniform(low=-0.5, high=1.5, size=parents.shape[1])
children[mutant] = children[mutant] + alpha*(ub-lb)
# 合并种群
population = np.vstack((population, children))
# 修剪种群
fitness = np.array([fitness_function(params) for params in population])
sorted_idx = np.argsort(fitness)[::-1]
population = population[sorted_idx[:n_crows]]
# 输出最优解
fitness = np.array([fitness_function(params) for params in population])
best_idx = np.argmax(fitness)
best_params = population[best_idx]
best_fitness = fitness[best_idx]
print('Best params:', best_params)
print('Best fitness:', best_fitness)
```
相关推荐
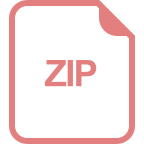














