小程序云开发在已完成的订单列表点击某一个订单的评价按钮跳转到评价页面,去填写评价,填写评价后返回订单列表,已评价的订单的评价按钮将不会显示示例及代码
时间: 2024-04-30 08:19:12 浏览: 84
这个功能可以通过以下步骤来实现:
1. 在订单列表页面中,为每个订单添加一个评价按钮,并为按钮绑定一个事件处理函数。在事件处理函数中,使用 `wx.navigateTo` 方法跳转到评价页面,并通过 URL 参数传递该订单的 ID。
2. 在评价页面中,通过 `wx.getStorageSync` 方法获取评价页面需要的数据,例如该订单的 ID、商品信息等。
3. 用户在评价页面填写完评价后,点击提交按钮,调用云函数将评价信息保存到云数据库中,并通过 `wx.navigateBack` 方法返回到订单列表页面。
4. 在订单列表页面的 `onShow` 事件处理函数中,重新加载订单列表数据,并根据每个订单的评价状态来判断是否需要显示评价按钮。
以下是示例代码:
订单列表页面:
```js
Page({
data: {
orders: []
},
onLoad() {
// 加载订单列表数据
this.loadOrders()
},
loadOrders() {
// 从云数据库中加载订单列表数据
wx.cloud.callFunction({
name: 'getOrders',
success: res => {
this.setData({
orders: res.result.data
})
}
})
},
onShow() {
// 重新加载订单列表数据
this.loadOrders()
},
onEvaluate(e) {
const orderId = e.currentTarget.dataset.orderId
// 跳转到评价页面
wx.navigateTo({
url: `/pages/evaluate/evaluate?orderId=${orderId}`
})
}
})
```
评价页面:
```js
Page({
data: {
orderId: '',
goods: [],
rating: 5,
comment: ''
},
onLoad(options) {
this.setData({
orderId: options.orderId
})
// 从本地缓存中获取商品信息
const goods = wx.getStorageSync('goods')
this.setData({
goods: goods
})
},
onRatingChange(e) {
this.setData({
rating: e.detail
})
},
onCommentChange(e) {
this.setData({
comment: e.detail
})
},
onSubmit() {
// 调用云函数保存评价信息
wx.cloud.callFunction({
name: 'evaluateOrder',
data: {
orderId: this.data.orderId,
rating: this.data.rating,
comment: this.data.comment
},
success: res => {
// 返回到订单列表页面
wx.navigateBack()
}
})
}
})
```
云函数:
```js
const cloud = require('wx-server-sdk')
cloud.init()
const db = cloud.database()
exports.main = async (event, context) => {
const { orderId, rating, comment } = event
try {
// 更新订单的评价状态和评价信息
await db.collection('orders').doc(orderId).update({
data: {
evaluated: true,
rating: rating,
comment: comment
}
})
return {
success: true
}
} catch (err) {
return {
success: false,
errMsg: err.message
}
}
}
```
阅读全文
相关推荐
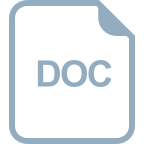
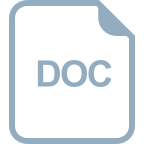
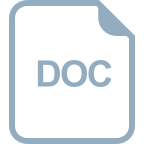


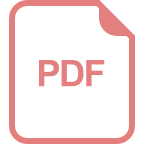
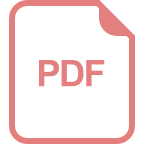
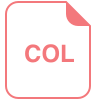








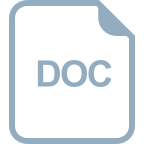
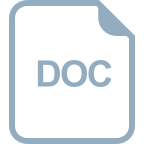