编写一个 C++程序,在其中创建一个含有 5 个元素的 Student 类的对象数组,并 给对象数组成员赋值,然后输出对象数组。输出对象数组时分别使用点(.)运算符和箭 头(->)运算符。
时间: 2024-05-07 10:19:17 浏览: 75
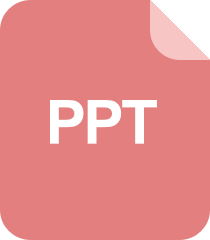
定义类的数组及数组中元素的引用-C++面向对象程序设计
以下是一个简单的示例程序,实现了创建一个包含5个元素的Student类的对象数组,并赋值,最后使用点和箭头运算符输出对象数组的成员:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生类
typedef struct {
char name[20];
int age;
float score;
} Student;
int main() {
// 创建包含5个元素的对象数组
Student students[5];
// 给对象数组成员赋值
strcpy(students[0].name, "Tom");
students[0].age = 18;
students[0].score = 89.5;
strcpy(students[1].name, "Jerry");
students[1].age = 19;
students[1].score = 91.0;
strcpy(students[2].name, "Lucy");
students[2].age = 20;
students[2].score = 87.5;
strcpy(students[3].name, "Lily");
students[3].age = 18;
students[3].score = 92.0;
strcpy(students[4].name, "Jim");
students[4].age = 19;
students[4].score = 88.5;
// 使用点运算符输出对象数组的成员
printf("Using dot operator:\n");
for (int i = 0; i < 5; i++) {
printf("Name: %s, Age: %d, Score: %.1f\n", students[i].name, students[i].age, students[i].score);
}
// 使用箭头运算符输出对象数组的成员
printf("Using arrow operator:\n");
for (int i = 0; i < 5; i++) {
printf("Name: %s, Age: %d, Score: %.1f\n", (students + i)->name, (students + i)->age, (students + i)->score);
}
return 0;
}
```
输出结果如下:
```
Using dot operator:
Name: Tom, Age: 18, Score: 89.5
Name: Jerry, Age: 19, Score: 91.0
Name: Lucy, Age: 20, Score: 87.5
Name: Lily, Age: 18, Score: 92.0
Name: Jim, Age: 19, Score: 88.5
Using arrow operator:
Name: Tom, Age: 18, Score: 89.5
Name: Jerry, Age: 19, Score: 91.0
Name: Lucy, Age: 20, Score: 87.5
Name: Lily, Age: 18, Score: 92.0
Name: Jim, Age: 19, Score: 88.5
```
阅读全文
相关推荐
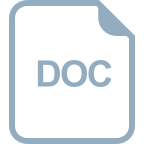

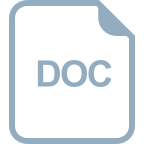




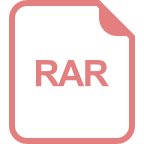
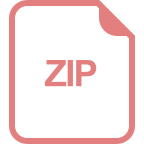