怎么向空的numpy数组中加入numpy数组
时间: 2023-09-08 08:14:23 浏览: 137
你可以使用numpy中的concatenate函数将两个numpy数组按照某个方向拼接起来,可以使用如下代码将一个numpy数组添加到另一个空的numpy数组中:
``` python
import numpy as np
# 空的numpy数组
empty_arr = np.empty([0, 3])
# 待添加的numpy数组
arr_to_add = np.array([[1, 2, 3], [4, 5, 6]])
# 在空的numpy数组中添加新的numpy数组
result = np.concatenate([empty_arr, arr_to_add])
```
当你运行这个代码时,你会得到一个形状为(2, 3)的numpy数组,其内容为:
```
[[1. 2. 3.]
[4. 5. 6.]]
```
希望这个回答能够帮到你!
相关问题
怎么向字典中加入numpy数组?
可以使用numpy数组的tolist()方法将其转换为Python列表,然后将列表添加到字典中。例如:
```
import numpy as np
my_dict = {'array': []}
my_array = np.array([1, 2, 3])
my_dict['array'] = my_array.tolist()
print(my_dict)
```
输出:
```
{'array': [1, 2, 3]}
```
在这个示例中,我们首先创建了一个空字典`my_dict`,然后创建一个numpy数组`my_array`。接下来,我们将numpy数组转换为Python列表,并将其添加到`my_dict`字典中的`array`键下。最后,我们打印出整个字典以确认数组已成功添加到字典中。
numpy数组如何分行加入元素
可以使用numpy的reshape函数将一维数组转为二维数组,然后再使用numpy的concatenate函数沿着列或行方向加入元素。例如:
import numpy as np
a = np.array([1, 2, 3, 4, 5, 6])
b = np.array([7, 8, 9, 10, 11, 12])
# 将一维数组转为二维数组
a = a.reshape(-1, 3)
b = b.reshape(-1, 3)
# 沿着行方向加入元素
c = np.concatenate((a, b), axis=0)
print(c)
输出结果为:
[[ 1 2 3]
[ 4 5 6]
[ 7 8 9]
[10 11 12]]
阅读全文
相关推荐
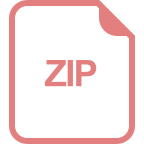
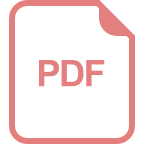
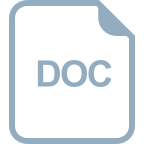
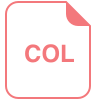









