利用 Java 开发一个可以进行简单的四则运算的图形化计算器,要求计算器至少能够实现基本的加减乘除运算以及实现括号功能。写出代码
时间: 2024-03-08 14:47:38 浏览: 80
好的,以下是一个简单的 Java 图形化计算器的代码,它可以实现基本的加减乘除运算以及实现括号功能:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Calculator extends JFrame implements ActionListener {
private JTextField inputField;
private JButton zeroBtn, oneBtn, twoBtn, threeBtn, fourBtn, fiveBtn, sixBtn, sevenBtn, eightBtn, nineBtn;
private JButton addBtn, subtractBtn, multiplyBtn, divideBtn, equalsBtn, clearBtn, openParenBtn, closeParenBtn;
private String currentInput = "";
public Calculator() {
setTitle("Calculator");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
inputField = new JTextField(20);
inputField.setEditable(false);
zeroBtn = new JButton("0");
oneBtn = new JButton("1");
twoBtn = new JButton("2");
threeBtn = new JButton("3");
fourBtn = new JButton("4");
fiveBtn = new JButton("5");
sixBtn = new JButton("6");
sevenBtn = new JButton("7");
eightBtn = new JButton("8");
nineBtn = new JButton("9");
addBtn = new JButton("+");
subtractBtn = new JButton("-");
multiplyBtn = new JButton("*");
divideBtn = new JButton("/");
equalsBtn = new JButton("=");
clearBtn = new JButton("C");
openParenBtn = new JButton("(");
closeParenBtn = new JButton(")");
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(4, 4));
buttonPanel.add(sevenBtn);
buttonPanel.add(eightBtn);
buttonPanel.add(nineBtn);
buttonPanel.add(divideBtn);
buttonPanel.add(fourBtn);
buttonPanel.add(fiveBtn);
buttonPanel.add(sixBtn);
buttonPanel.add(multiplyBtn);
buttonPanel.add(oneBtn);
buttonPanel.add(twoBtn);
buttonPanel.add(threeBtn);
buttonPanel.add(subtractBtn);
buttonPanel.add(zeroBtn);
buttonPanel.add(openParenBtn);
buttonPanel.add(closeParenBtn);
buttonPanel.add(addBtn);
JPanel clearPanel = new JPanel();
clearPanel.add(clearBtn);
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
contentPane.add(inputField, BorderLayout.NORTH);
contentPane.add(buttonPanel, BorderLayout.CENTER);
contentPane.add(clearPanel, BorderLayout.SOUTH);
zeroBtn.addActionListener(this);
oneBtn.addActionListener(this);
twoBtn.addActionListener(this);
threeBtn.addActionListener(this);
fourBtn.addActionListener(this);
fiveBtn.addActionListener(this);
sixBtn.addActionListener(this);
sevenBtn.addActionListener(this);
eightBtn.addActionListener(this);
nineBtn.addActionListener(this);
addBtn.addActionListener(this);
subtractBtn.addActionListener(this);
multiplyBtn.addActionListener(this);
divideBtn.addActionListener(this);
equalsBtn.addActionListener(this);
clearBtn.addActionListener(this);
openParenBtn.addActionListener(this);
closeParenBtn.addActionListener(this);
pack();
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == zeroBtn) {
currentInput += "0";
} else if (e.getSource() == oneBtn) {
currentInput += "1";
} else if (e.getSource() == twoBtn) {
currentInput += "2";
} else if (e.getSource() == threeBtn) {
currentInput += "3";
} else if (e.getSource() == fourBtn) {
currentInput += "4";
} else if (e.getSource() == fiveBtn) {
currentInput += "5";
} else if (e.getSource() == sixBtn) {
currentInput += "6";
} else if (e.getSource() == sevenBtn) {
currentInput += "7";
} else if (e.getSource() == eightBtn) {
currentInput += "8";
} else if (e.getSource() == nineBtn) {
currentInput += "9";
} else if (e.getSource() == addBtn) {
currentInput += "+";
} else if (e.getSource() == subtractBtn) {
currentInput += "-";
} else if (e.getSource() == multiplyBtn) {
currentInput += "*";
} else if (e.getSource() == divideBtn) {
currentInput += "/";
} else if (e.getSource() == openParenBtn) {
currentInput += "(";
} else if (e.getSource() == closeParenBtn) {
currentInput += ")";
} else if (e.getSource() == equalsBtn) {
try {
String result = evaluate(currentInput);
inputField.setText(result);
currentInput = "";
} catch (Exception ex) {
inputField.setText("Error");
currentInput = "";
}
} else if (e.getSource() == clearBtn) {
inputField.setText("");
currentInput = "";
}
inputField.setText(currentInput);
}
public static String evaluate(String expression) {
char[] tokens = expression.toCharArray();
Stack<Integer> values = new Stack<Integer>();
Stack<Character> ops = new Stack<Character>();
for (int i = 0; i < tokens.length; i++) {
if (tokens[i] == ' ')
continue;
if (tokens[i] >= '0' && tokens[i] <= '9') {
StringBuffer sbuf = new StringBuffer();
while (i < tokens.length && tokens[i] >= '0' && tokens[i] <= '9')
sbuf.append(tokens[i++]);
values.push(Integer.parseInt(sbuf.toString()));
}
else if (tokens[i] == '(')
ops.push(tokens[i]);
else if (tokens[i] == ')') {
while (ops.peek() != '(')
values.push(applyOp(ops.pop(), values.pop(), values.pop()));
ops.pop();
}
else if (tokens[i] == '+' || tokens[i] == '-' || tokens[i] == '*' || tokens[i] == '/') {
while (!ops.empty() && hasPrecedence(tokens[i], ops.peek()))
values.push(applyOp(ops.pop(), values.pop(), values.pop()));
ops.push(tokens[i]);
}
}
while (!ops.empty())
values.push(applyOp(ops.pop(), values.pop(), values.pop()));
return Integer.toString(values.pop());
}
public static boolean hasPrecedence(char op1, char op2) {
if (op2 == '(' || op2 == ')')
return false;
if ((op1 == '*' || op1 == '/') && (op2 == '+' || op2 == '-'))
return false;
else
return true;
}
public static int applyOp(char op, int b, int a) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
if (b == 0)
throw new UnsupportedOperationException("Cannot divide by zero");
return a / b;
}
return 0;
}
public static void main(String[] args) {
new Calculator();
}
}
```
希望这个例子能够帮助你实现自己的图形化计算器。
阅读全文
相关推荐




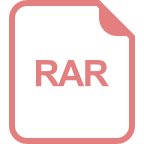


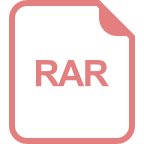








