C++二维图像扶正
时间: 2023-07-04 11:22:10 浏览: 49
这里提供一种简单的C++实现方法,基于OpenCV库实现,具体步骤如下:
1. 安装OpenCV库,并导入头文件。
```c++
#include <opencv2/opencv.hpp>
using namespace cv;
```
2. 读取需要扶正的图像,并将其转换为灰度图像。
```c++
Mat img = imread("input.jpg");
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
```
3. 使用霍夫变换检测图像中的直线特征。
```c++
vector<Vec2f> lines;
HoughLines(gray, lines, 1, CV_PI / 180, 100, 0, 0);
```
4. 根据直线的位置和方向,计算需要旋转的角度。
```c++
float angle = 0.0;
for (size_t i = 0; i < lines.size(); i++) {
float rho = lines[i][0], theta = lines[i][1];
if (theta < CV_PI / 4. || theta > 3.*CV_PI / 4.) {
angle = theta;
break;
}
}
```
5. 对图像进行旋转,将直线旋转到水平方向。
```c++
Mat rotated;
Point2f pt(img.cols / 2., img.rows / 2.);
Mat r = getRotationMatrix2D(pt, angle * 180 / CV_PI, 1.0);
warpAffine(img, rotated, r, img.size());
```
6. 根据需要,进行图像的裁剪或者填充操作,得到最终的扶正图像。
```c++
Mat result;
if (rotated.cols > rotated.rows) {
int y = (rotated.cols - rotated.rows) / 2;
result = rotated(Rect(y, 0, rotated.rows, rotated.rows));
}
else {
int x = (rotated.rows - rotated.cols) / 2;
result = rotated(Rect(0, x, rotated.cols, rotated.cols));
}
```
完整的代码如下:
```c++
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
Mat img = imread("input.jpg");
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
vector<Vec2f> lines;
HoughLines(gray, lines, 1, CV_PI / 180, 100, 0, 0);
float angle = 0.0;
for (size_t i = 0; i < lines.size(); i++) {
float rho = lines[i][0], theta = lines[i][1];
if (theta < CV_PI / 4. || theta > 3.*CV_PI / 4.) {
angle = theta;
break;
}
}
Mat rotated;
Point2f pt(img.cols / 2., img.rows / 2.);
Mat r = getRotationMatrix2D(pt, angle * 180 / CV_PI, 1.0);
warpAffine(img, rotated, r, img.size());
Mat result;
if (rotated.cols > rotated.rows) {
int y = (rotated.cols - rotated.rows) / 2;
result = rotated(Rect(y, 0, rotated.rows, rotated.rows));
}
else {
int x = (rotated.rows - rotated.cols) / 2;
result = rotated(Rect(0, x, rotated.cols, rotated.cols));
}
imshow("input", img);
imshow("output", result);
waitKey();
return 0;
}
```
需要注意的是,上述代码中的参数都是示例值,需要根据具体情况进行调整。同时,由于上述算法依赖于直线特征的检测,因此如果图像中没有明显的直线特征,可能无法得到准确的扶正结果。
相关推荐
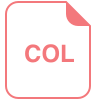
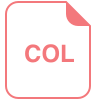
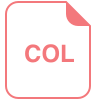
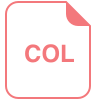
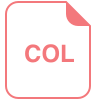









