@GetMapping("/bookIndex") public String bookIndex(){ return "book/bookIndex"; }
时间: 2024-04-20 07:22:53 浏览: 23
这段代码是一个使用Spring MVC框架的Java控制器方法。该方法使用@GetMapping注解来处理GET请求,其URL路径为"/bookIndex"。
在方法体内部,该方法直接返回一个字符串"book/bookIndex"。根据返回的字符串,可以推断出该方法用于返回一个名为"bookIndex"的视图页面。
需要注意的是,返回的字符串并不是直接返回给客户端,而是作为逻辑视图名称,Spring MVC会根据该名称解析对应的视图模板,并将其渲染后返回给客户端。这样可以实现页面跳转或者返回特定的视图页面。
相关问题
springboot实现图书管理系统过程例子
好的,下面是一个简单的基于Spring Boot实现的图书管理系统的例子,包含基本的增删改查功能。
1. 创建一个Spring Boot项目,引入相关依赖。
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
```
2. 创建一个图书实体类Book,使用JPA注解标识实体属性和关系。
```java
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String title;
private String author;
private String description;
// getter/setter
}
```
3. 创建一个BookRepository接口,继承JpaRepository接口,用于操作数据库。
```java
public interface BookRepository extends JpaRepository<Book, Long> {
}
```
4. 创建一个BookController类,用于处理请求和返回视图。
```java
@Controller
public class BookController {
@Autowired
private BookRepository bookRepository;
@GetMapping("/")
public String index(Model model) {
List<Book> books = bookRepository.findAll();
model.addAttribute("books", books);
return "index";
}
@GetMapping("/add")
public String addForm(Model model) {
model.addAttribute("book", new Book());
return "add";
}
@PostMapping("/add")
public String addSubmit(@ModelAttribute Book book) {
bookRepository.save(book);
return "redirect:/";
}
@GetMapping("/edit/{id}")
public String editForm(@PathVariable Long id, Model model) {
Book book = bookRepository.findById(id).orElseThrow(() -> new IllegalArgumentException("Invalid book id:" + id));
model.addAttribute("book", book);
return "edit";
}
@PostMapping("/edit/{id}")
public String editSubmit(@PathVariable Long id, @ModelAttribute Book book) {
book.setId(id);
bookRepository.save(book);
return "redirect:/";
}
@GetMapping("/delete/{id}")
public String delete(@PathVariable Long id) {
bookRepository.deleteById(id);
return "redirect:/";
}
}
```
5. 创建Thymeleaf模板,用于展示图书信息和表单。
index.html
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>图书管理系统</title>
</head>
<body>
<h1>图书列表</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>书名</th>
<th>作者</th>
<th>简介</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="book : ${books}">
<td th:text="${book.id}"></td>
<td th:text="${book.title}"></td>
<td th:text="${book.author}"></td>
<td th:text="${book.description}"></td>
<td>
<a th:href="@{/edit/{id}(id=${book.id})}">编辑</a>
<a th:href="@{/delete/{id}(id=${book.id})}">删除</a>
</td>
</tr>
</tbody>
</table>
<br>
<a th:href="@{/add}">添加图书</a>
</body>
</html>
```
add.html
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>添加图书</title>
</head>
<body>
<h1>添加图书</h1>
<form th:action="@{/add}" th:object="${book}" method="post">
<table>
<tr>
<td>书名:</td>
<td><input type="text" th:field="*{title}"></td>
</tr>
<tr>
<td>作者:</td>
<td><input type="text" th:field="*{author}"></td>
</tr>
<tr>
<td>简介:</td>
<td><textarea th:field="*{description}"></textarea></td>
</tr>
<tr>
<td></td>
<td><input type="submit" value="添加"></td>
</tr>
</table>
</form>
</body>
</html>
```
edit.html
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>编辑图书</title>
</head>
<body>
<h1>编辑图书</h1>
<form th:action="@{/edit/{id}(id=${book.id})}" th:object="${book}" method="post">
<table>
<tr>
<td>书名:</td>
<td><input type="text" th:field="*{title}"></td>
</tr>
<tr>
<td>作者:</td>
<td><input type="text" th:field="*{author}"></td>
</tr>
<tr>
<td>简介:</td>
<td><textarea th:field="*{description}"></textarea></td>
</tr>
<tr>
<td></td>
<td><input type="submit" value="保存"></td>
</tr>
</table>
</form>
</body>
</html>
```
6. 启动应用,访问http://localhost:8080/,可以看到图书列表,点击添加图书按钮,可以添加一本新图书,点击编辑按钮,可以修改图书信息,点击删除按钮,可以删除图书。
以上就是一个简单的基于Spring Boot实现的图书管理系统例子,希望能对你有所帮助。
用springboot写一个图书管理系统
好的,下面是一个简单的图书管理系统的实现,使用了Spring Boot框架和MySQL数据库。
首先,我们需要创建一个Spring Boot项目。在pom.xml文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- MySQL Connector -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!-- Spring Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- Thymeleaf -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
```
接下来,我们需要创建一个Book实体类,用于表示图书信息,代码如下:
```java
@Entity
@Table(name = "books")
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String title;
@Column(nullable = false)
private String author;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
}
```
然后,我们需要创建一个BookRepository接口,用于对图书信息进行增删改查操作,代码如下:
```java
public interface BookRepository extends JpaRepository<Book, Long> {
List<Book> findByTitleContainingOrAuthorContaining(String keyword1, String keyword2);
}
```
接下来,我们需要创建一个BookController类,用于处理图书信息的请求和响应,代码如下:
```java
@Controller
public class BookController {
@Autowired
private BookRepository bookRepository;
@GetMapping("/")
public String index(Model model) {
List<Book> books = bookRepository.findAll();
model.addAttribute("books", books);
return "index";
}
@GetMapping("/search")
public String search(@RequestParam("q") String query, Model model) {
List<Book> books = bookRepository.findByTitleContainingOrAuthorContaining(query, query);
model.addAttribute("books", books);
return "search";
}
@GetMapping("/add")
public String add(Model model) {
model.addAttribute("book", new Book());
return "add";
}
@PostMapping("/add")
public String add(@ModelAttribute("book") Book book) {
bookRepository.save(book);
return "redirect:/";
}
@GetMapping("/edit/{id}")
public String edit(@PathVariable("id") Long id, Model model) {
Optional<Book> book = bookRepository.findById(id);
if (book.isPresent()) {
model.addAttribute("book", book.get());
return "edit";
} else {
return "redirect:/";
}
}
@PostMapping("/edit")
public String edit(@ModelAttribute("book") Book book) {
bookRepository.save(book);
return "redirect:/";
}
@GetMapping("/delete/{id}")
public String delete(@PathVariable("id") Long id) {
bookRepository.deleteById(id);
return "redirect:/";
}
}
```
最后,我们需要创建一些HTML模板文件,用于显示图书信息和处理用户的请求,代码如下:
index.html:
```html
<!DOCTYPE html>
<html>
<head>
<title>Book Management System</title>
</head>
<body>
<h1>Book Management System</h1>
<form action="/search" method="get">
<input type="text" name="q" placeholder="Search...">
<button type="submit">Search</button>
</form>
<table>
<tr>
<th>ID</th>
<th>Title</th>
<th>Author</th>
<th>Action</th>
</tr>
<tr th:each="book : ${books}">
<td th:text="${book.id}"></td>
<td th:text="${book.title}"></td>
<td th:text="${book.author}"></td>
<td>
<a th:href="@{/edit/{id}(id=${book.id})}">Edit</a>
<a th:href="@{/delete/{id}(id=${book.id})}" onclick="return confirm('Are you sure?')">Delete</a>
</td>
</tr>
</table>
<br>
<a href="/add">Add New Book</a>
</body>
</html>
```
search.html:
```html
<!DOCTYPE html>
<html>
<head>
<title>Book Management System</title>
</head>
<body>
<h1>Book Management System</h1>
<form action="/search" method="get">
<input type="text" name="q" placeholder="Search..." value="${param.q}">
<button type="submit">Search</button>
</form>
<table>
<tr>
<th>ID</th>
<th>Title</th>
<th>Author</th>
<th>Action</th>
</tr>
<tr th:each="book : ${books}">
<td th:text="${book.id}"></td>
<td th:text="${book.title}"></td>
<td th:text="${book.author}"></td>
<td>
<a th:href="@{/edit/{id}(id=${book.id})}">Edit</a>
<a th:href="@{/delete/{id}(id=${book.id})}" onclick="return confirm('Are you sure?')">Delete</a>
</td>
</tr>
</table>
<br>
<a href="/add">Add New Book</a>
</body>
</html>
```
add.html:
```html
<!DOCTYPE html>
<html>
<head>
<title>Book Management System</title>
</head>
<body>
<h1>Book Management System</h1>
<form action="/add" method="post">
<label>Title:</label><br>
<input type="text" name="title"><br>
<label>Author:</label><br>
<input type="text" name="author"><br>
<button type="submit">Add Book</button>
</form>
<br>
<a href="/">Back to Home</a>
</body>
</html>
```
edit.html:
```html
<!DOCTYPE html>
<html>
<head>
<title>Book Management System</title>
</head>
<body>
<h1>Book Management System</h1>
<form action="/edit" method="post">
<input type="hidden" name="id" th:value="${book.id}">
<label>Title:</label><br>
<input type="text" name="title" th:value="${book.title}"><br>
<label>Author:</label><br>
<input type="text" name="author" th:value="${book.author}"><br>
<button type="submit">Save Changes</button>
</form>
<br>
<a href="/">Back to Home</a>
</body>
</html>
```
这样,一个简单的图书管理系统就完成了。启动应用后,访问 http://localhost:8080/ 即可进入主界面,可以进行图书信息的增删改查操作。
相关推荐
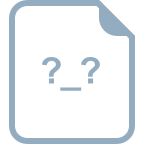
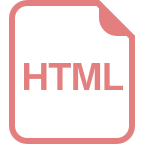
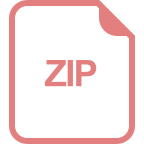










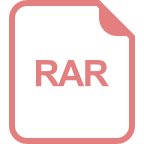
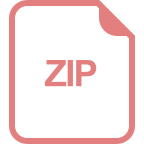