组件写简易分页器
时间: 2023-07-08 14:09:21 浏览: 103
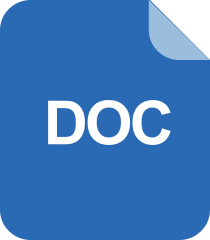
S2SH项目中的分页组件

好的,下面是一个简易的分页器组件实现:
```html
<template>
<div class="pagination">
<button :disabled="currentPage === 1" @click="changePage(1)">首页</button>
<button :disabled="currentPage === 1" @click="changePage(currentPage - 1)">上一页</button>
<span v-for="page in pages" :key="page" :class="{ active: currentPage === page }" @click="changePage(page)">{{ page }}</span>
<button :disabled="currentPage === totalPage" @click="changePage(currentPage + 1)">下一页</button>
<button :disabled="currentPage === totalPage" @click="changePage(totalPage)">末页</button>
</div>
</template>
<script>
export default {
name: "Pagination",
props: {
currentPage: {
type: Number,
required: true
},
totalPage: {
type: Number,
required: true
}
},
computed: {
pages() {
let start, end;
if (this.totalPage <= 5) {
start = 1;
end = this.totalPage;
} else {
if (this.currentPage <= 3) {
start = 1;
end = 5;
} else if (this.currentPage >= this.totalPage - 2) {
start = this.totalPage - 4;
end = this.totalPage;
} else {
start = this.currentPage - 2;
end = this.currentPage + 2;
}
}
return Array.from({ length: end - start + 1 }, (_, i) => start + i);
}
},
methods: {
changePage(page) {
if (page !== this.currentPage) {
this.$emit("change", page);
}
}
}
};
</script>
<style>
.pagination {
display: flex;
justify-content: center;
align-items: center;
margin: 20px 0;
}
button,
span {
margin: 0 5px;
padding: 5px 10px;
border: none;
border-radius: 5px;
cursor: pointer;
}
button[disabled],
span.active {
background-color: #ccc;
cursor: default;
}
</style>
```
使用示例:
```vue
<template>
<div>
<div v-for="item in items" :key="item.id">{{ item.name }}</div>
<pagination :current-page="currentPage" :total-page="totalPage" @change="handlePageChange" />
</div>
</template>
<script>
import Pagination from "@/components/Pagination.vue";
export default {
name: "ItemList",
components: {
Pagination
},
data() {
return {
items: [],
currentPage: 1,
totalPage: 1
};
},
mounted() {
this.fetchData();
},
methods: {
fetchData() {
// 发送请求获取数据并更新items、totalPage等数据
},
handlePageChange(page) {
this.currentPage = page;
this.fetchData();
}
}
};
</script>
```
这个分页器组件实现了基本的分页功能,包括首页、上一页、下一页、末页和页码列表。其中,页码列表根据当前页码和总页数动态生成,最多显示5个页码,当前页码高亮显示。组件通过 `props` 接收当前页码和总页数,通过 `$emit` 事件传递页码变化的消息。在使用时,只需要在父组件中监听 `change` 事件,更新当前页码并重新获取数据即可。
阅读全文
相关推荐
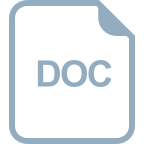
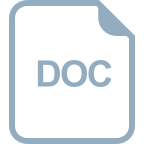




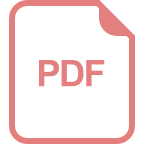
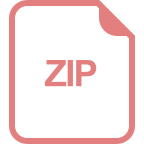
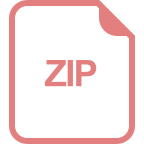
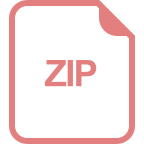
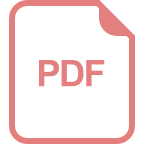
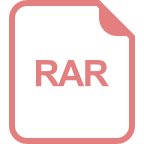
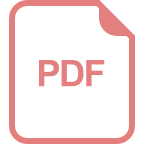
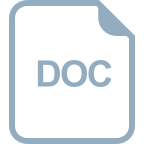
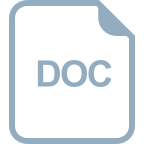
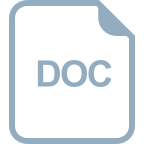

