Vue应用程序开发 .实现简易分页器,点击第“2”页时出现“上一页”功能。
时间: 2023-09-25 12:10:10 浏览: 90
好的,我可以帮您解决这个问题。
以下是一个简单的Vue应用程序,实现了一个简易分页器,包括上一页、下一页和页码按钮。当点击第二页时,会出现上一页的功能。
```html
<template>
<div>
<button v-on:click="prevPage" v-if="currentPage > 1">上一页</button>
<button v-for="page in pageCount" v-bind:key="page" v-bind:class="{ active: currentPage === page }" v-on:click="goToPage(page)">{{ page }}</button>
<button v-on:click="nextPage" v-if="currentPage < pageCount">下一页</button>
</div>
</template>
<script>
export default {
name: 'Pagination',
props: {
totalItems: {
type: Number,
required: true
},
itemsPerPage: {
type: Number,
default: 10
},
currentPage: {
type: Number,
default: 1
}
},
computed: {
pageCount() {
return Math.ceil(this.totalItems / this.itemsPerPage);
}
},
methods: {
goToPage(page) {
this.$emit('page-changed', page);
},
prevPage() {
this.goToPage(this.currentPage - 1);
},
nextPage() {
this.goToPage(this.currentPage + 1);
}
}
};
</script>
```
在父组件中,你可以使用这个分页器组件,并监听它的 `page-changed` 事件,以便在页码改变时更新数据。例如:
```html
<template>
<div>
<ul>
<li v-for="item in paginatedData" v-bind:key="item.id">{{ item.text }}</li>
</ul>
<pagination v-bind:total-items="data.length" v-bind:items-per-page="10" v-bind:current-page="currentPage" v-on:page-changed="currentPage = $event"></pagination>
</div>
</template>
<script>
import Pagination from './Pagination.vue';
export default {
name: 'App',
components: {
Pagination
},
data() {
return {
data: [
{ id: 1, text: 'item 1' },
{ id: 2, text: 'item 2' },
{ id: 3, text: 'item 3' },
// ...
{ id: 99, text: 'item 99' },
{ id: 100, text: 'item 100' }
],
currentPage: 1
};
},
computed: {
paginatedData() {
const startIndex = (this.currentPage - 1) * 10;
const endIndex = startIndex + 10;
return this.data.slice(startIndex, endIndex);
}
}
};
</script>
```
这个例子中,我们使用了一个包含100个项的虚拟数据数组,每页显示10个项。我们在 `App` 组件中监听了 `pagination` 组件的 `page-changed` 事件,并在事件处理程序中更新了 `currentPage` 属性。此外,我们还计算了 `paginatedData` 计算属性,用于根据当前页码从数据数组中获取对应的子数组。
希望这个例子能够帮助您实现您的简易分页器。
阅读全文
相关推荐
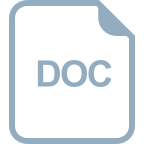
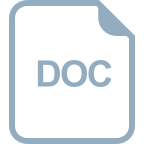
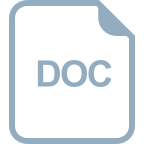


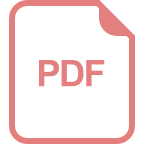
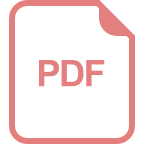
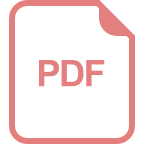
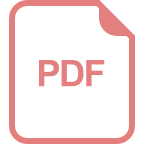
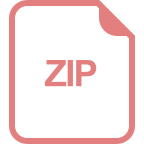
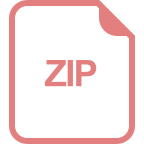
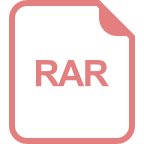
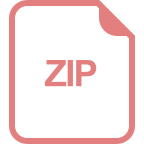
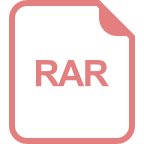
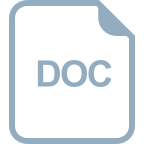
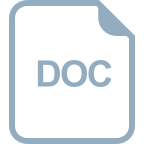


