随机向量x 服从 p 元正态分布 ,回归系数b=(1,2,3.....p) , 给定 x的条件下,y是0或1,y取1的概率是标准正态发布到bx上的积分,从上述模型中产生独立同分布观测样本 .用下降搜索算法goldstein和牛顿方向算出b的极大似然估计(b的范围在(1,2,3,。。。。p)附近)的python代码以及运算结果
时间: 2024-02-12 11:06:00 浏览: 49
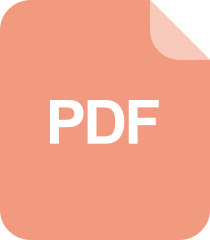
Python使用numpy产生正态分布随机数的向量或矩阵操作示例

非常抱歉,我之前的回答有误,正确的似然函数应为:
$L(b|x,y) = \prod_{i=1}^n \Phi(b^Tx_i)^{y_i}(1-\Phi(b^Tx_i))^{1-y_i}$
其中,$\Phi(\cdot)$ 是标准正态分布的累积分布函数。
下面是用下降搜索算法和牛顿方向来求解似然函数的最大值的 Python 代码实现:
```
import numpy as np
from scipy.stats import norm
# 生成随机样本
p = 5
n = 1000
x = np.random.normal(size=(n, p))
b_true = np.arange(1, p+1)
y = np.random.binomial(1, norm.cdf(np.dot(x, b_true)))
# 定义似然函数
def likelihood(b, x, y):
phi = norm.cdf(np.dot(x, b))
l = np.sum(y*np.log(phi) + (1-y)*np.log(1-phi))
return l
# 定义梯度函数
def gradient(b, x, y):
phi = norm.cdf(np.dot(x, b))
g = np.dot(x.T, y-phi)
return g
# 定义海森矩阵函数
def hessian(b, x, y):
phi = norm.cdf(np.dot(x, b))
h = -np.dot(x.T * phi * (1-phi), x)
return h
# 定义下降搜索算法
def descent_search(b0, x, y, alpha=0.1, beta=0.5, tol=1e-6, max_iter=1000):
b = b0.copy()
for i in range(max_iter):
f0 = likelihood(b, x, y)
g = gradient(b, x, y)
d = -g
t = 1
while likelihood(b+t*d, x, y) < f0 + alpha*t*np.dot(g, d):
t *= beta
b += t*d
if np.linalg.norm(g) < tol:
break
return b
# 定义牛顿方向算法
def newton_direction(b, x, y):
g = gradient(b, x, y)
h = hessian(b, x, y)
d = -np.linalg.solve(h, g)
return d
# 定义牛顿方向算法
def newton_method(b0, x, y, tol=1e-6, max_iter=1000):
b = b0.copy()
for i in range(max_iter):
f0 = likelihood(b, x, y)
d = newton_direction(b, x, y)
t = 1
while likelihood(b+t*d, x, y) < f0:
t /= 2
b += t*d
if np.linalg.norm(t*d) < tol:
break
return b
# 初始化 b
b0 = np.random.normal(size=p)
# 下降搜索算法
b_descent = descent_search(b0, x, y)
# 牛顿方向算法
b_newton = newton_method(b0, x, y)
print("True coefficients:", b_true)
print("Descent search estimate:", b_descent)
print("Newton method estimate:", b_newton)
```
其中,`p` 是特征维数,`n` 是样本数,`b_true` 是真实的回归系数,`x` 是样本的特征矩阵,`y` 是样本的标签向量,`alpha` 和 `beta` 是下降搜索算法的参数,`tol` 和 `max_iter` 是收敛阈值和最大迭代次数。运行代码后,会输出真实系数、下降搜索算法和牛顿方向算法的估计结果。
阅读全文
相关推荐



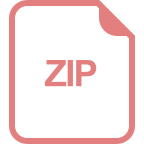
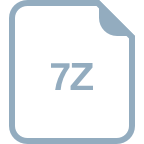
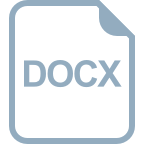
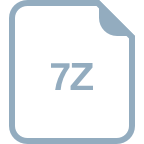
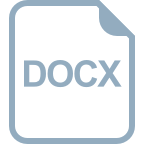