#include<iostream> using namespace std; //创建一个点类 class point { public: void setx(int a) { x = a; }//设置x int getx() { return x; }//获取x void sety(int b) { y = b; }//设置y int gety() { return y; }//获取y private: int x; int y; }; class circle//创建一个圆类 { public: void setr(int r) { c_R = r; }//设置半径 int getr() { return c_R; }//获取半径 void setpoint(point q) { p = q; }//设置圆心 point getpoint() { return p; }//获取圆心 private: int c_R; point p; }; //判断点与圆的关系 void oncircle(circle& c, point &p) { //先算点到圆心的距离de平方 int distance = (p.getx() - c.getpoint().getx) * (p.getx() - c.getpoint().getx) + (p.gety() - c.getpoint().gety) * (p.gety() - c.getpoint().gety); } void main() { point a,o; o.setx(10); o.sety(20); a.setx(15); a.sety(18); circle C; C.setr(10); C.setpoint(o); system("pause"); }以上代码哪里错了
时间: 2024-02-05 09:05:12 浏览: 32
代码中有一个错误,即在 `oncircle()` 函数中获取圆心的 x 坐标时漏掉了括号,应该写成 `c.getpoint().getx()`。因此,正确的代码应该是:
```
#include<iostream>
using namespace std;
//创建一个点类
class point {
public:
void setx(int a)
{
x = a;
}//设置x
int getx()
{
return x;
}//获取x
void sety(int b)
{
y = b;
}//设置y
int gety()
{
return y;
}//获取y
private:
int x;
int y;
};
//创建一个圆类
class circle {
public:
void setr(int r)
{
c_R = r;
}//设置半径
int getr()
{
return c_R;
}//获取半径
void setpoint(point q)
{
p = q;
}//设置圆心
point getpoint()
{
return p;
}//获取圆心
private:
int c_R;
point p;
};
//判断点与圆的关系
void oncircle(circle& c, point &p) {
//先算点到圆心的距离de平方
int distance =
(p.getx() - c.getpoint().getx()) * (p.getx() - c.getpoint().getx())
+ (p.gety() - c.getpoint().gety()) * (p.gety() - c.getpoint().gety);
}
void main() {
point a,o;
o.setx(10);
o.sety(20);
a.setx(15);
a.sety(18);
circle C;
C.setr(10);
C.setpoint(o);
system("pause");
}
```
相关推荐
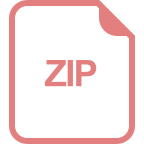
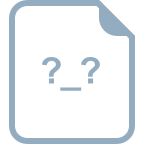















