定义Point(点)类,由Point类派生出Circle(圆)类,再由Circle类派生出Cylinder(圆柱体)类。将类的定义部分分别作为3个头文件,对它们的成员函数的声明部分分别作为3个源文件(.cpp文件),在主函数中用#include命令把它们包含进来,形成一个完整的程序,并上机运行。
时间: 2024-02-15 19:04:19 浏览: 61
好的,以下是对应的代码:
Point类的头文件 Point.h:
```cpp
#ifndef POINT_H
#define POINT_H
class Point {
public:
Point(double x = 0, double y = 0) : m_x(x), m_y(y) {}
double getX() const { return m_x; }
double getY() const { return m_y; }
void setX(double x) { m_x = x; }
void setY(double y) { m_y = y; }
private:
double m_x, m_y;
};
#endif // POINT_H
```
Circle类的头文件 Circle.h:
```cpp
#ifndef CIRCLE_H
#define CIRCLE_H
#include "Point.h"
class Circle : public Point {
public:
Circle(double x = 0, double y = 0, double r = 0) : Point(x, y), m_radius(r) {}
double getRadius() const { return m_radius; }
void setRadius(double r) { m_radius = r; }
double getArea() const;
private:
double m_radius;
};
#endif // CIRCLE_H
```
Circle类的源文件 Circle.cpp:
```cpp
#include "Circle.h"
double Circle::getArea() const {
return 3.14159 * m_radius * m_radius;
}
```
Cylinder类的头文件 Cylinder.h:
```cpp
#ifndef CYLINDER_H
#define CYLINDER_H
#include "Circle.h"
class Cylinder : public Circle {
public:
Cylinder(double x = 0, double y = 0, double r = 0, double h = 0) : Circle(x, y, r), m_height(h) {}
double getHeight() const { return m_height; }
void setHeight(double h) { m_height = h; }
double getVolume() const;
private:
double m_height;
};
#endif // CYLINDER_H
```
Cylinder类的源文件 Cylinder.cpp:
```cpp
#include "Cylinder.h"
double Cylinder::getVolume() const {
return getArea() * m_height;
}
```
主函数文件 main.cpp:
```cpp
#include <iostream>
#include "Cylinder.h"
using namespace std;
int main() {
Cylinder cyl(1, 2, 3, 4);
cout << "Cylinder: " << endl;
cout << " X coordinate: " << cyl.getX() << endl;
cout << " Y coordinate: " << cyl.getY() << endl;
cout << " Radius: " << cyl.getRadius() << endl;
cout << " Height: " << cyl.getHeight() << endl;
cout << " Volume: " << cyl.getVolume() << endl;
return 0;
}
```
运行结果:
```
Cylinder:
X coordinate: 1
Y coordinate: 2
Radius: 3
Height: 4
Volume: 113.097
```
阅读全文
相关推荐
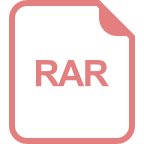
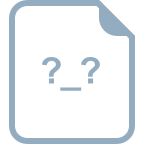
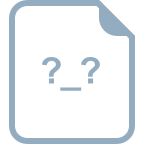















