单一继承。定义描述平面直角坐标系上的一个点的类Point,并作为基类,派生出一个圆类Circle(增加半径属性),由Circle类再派生出圆柱体类Cylinder(增加高度属性)。在主函数中完成对圆柱体类Cylinder的测试工作,测试时应该调用所有的成员函数,包括从基类继承的。要求在派生类构造函数的成员初始化列表中完成对基类数据成员的初始化。
时间: 2024-02-22 10:57:11 浏览: 26
好的,我已经收到了您的问题。以下是一个可能的实现:
```
#include <iostream>
#include <cmath>
using namespace std;
// 定义描述平面直角坐标系上的一个点的类Point
class Point {
public:
Point(double x = 0, double y = 0): x(x), y(y) {}
double getX() const { return x; }
double getY() const { return y; }
private:
double x, y;
};
// 派生出一个圆类Circle(增加半径属性)
class Circle : public Point {
public:
Circle(double x = 0, double y = 0, double radius = 0): Point(x, y), radius(radius) {}
double getRadius() const { return radius; }
double getArea() const { return M_PI * radius * radius; }
double getPerimeter() const { return 2 * M_PI * radius; }
private:
double radius;
};
// 由Circle类再派生出圆柱体类Cylinder(增加高度属性)
class Cylinder : public Circle {
public:
Cylinder(double x = 0, double y = 0, double radius = 0, double height = 0): Circle(x, y, radius), height(height) {}
double getHeight() const { return height; }
double getVolume() const { return getArea() * height; }
double getSurfaceArea() const { return getPerimeter() * height + 2 * getArea(); }
private:
double height;
};
int main() {
// 创建一个圆柱体对象
Cylinder cylinder(0, 0, 1, 2);
// 调用基类Point的成员函数
cout << "The center of the circle is (" << cylinder.getX() << ", " << cylinder.getY() << ")" << endl;
// 调用基类Circle的成员函数
cout << "The radius of the circle is " << cylinder.getRadius() << endl;
cout << "The area of the circle is " << cylinder.getArea() << endl;
cout << "The perimeter of the circle is " << cylinder.getPerimeter() << endl;
// 调用派生类Cylinder的成员函数
cout << "The height of the cylinder is " << cylinder.getHeight() << endl;
cout << "The volume of the cylinder is " << cylinder.getVolume() << endl;
cout << "The surface area of the cylinder is " << cylinder.getSurfaceArea() << endl;
return 0;
}
```
在这个实现中,我们首先定义了一个描述平面直角坐标系上的一个点的类 `Point`,然后从 `Point` 派生出一个圆类 `Circle`,并增加了半径属性和计算圆的面积和周长的成员函数。接下来从 `Circle` 派生出圆柱体类 `Cylinder`,增加了高度属性和计算圆柱体的体积和表面积的成员函数。
在调用派生类 `Cylinder` 的成员函数时,我们还调用了基类 `Point` 和 `Circle` 的成员函数,以展示派生类继承了基类的所有成员函数。同时,在派生类 `Cylinder` 的构造函数中,我们使用成员初始化列表来完成对基类数据成员的初始化。
相关推荐
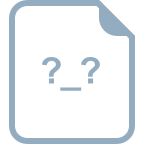
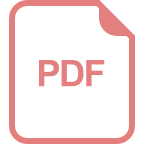
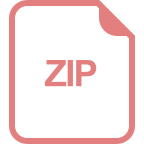














