定义一个类,由point类派生出circle类(圆),由circle类派生出cylinder类(圆柱体)。将类的定义部分分别做3个头文件,对它们的成员函数的声明与实现分别作为3个源文件。对其成员函数的声音部分分别作为3个不同的源文件做成3个源文件。
时间: 2024-03-17 18:44:45 浏览: 78
好的,这是您要求的类的定义,分别存放在三个头文件中。
point.h:
```cpp
#ifndef POINT_H
#define POINT_H
class Point {
public:
Point(double x = 0, double y = 0);
void setX(double x);
double getX() const;
void setY(double y);
double getY() const;
private:
double x, y;
};
#endif
```
circle.h:
```cpp
#ifndef CIRCLE_H
#define CIRCLE_H
#include "point.h"
class Circle : public Point {
public:
Circle(double x = 0, double y = 0, double r = 0);
void setR(double r);
double getR() const;
double getArea() const;
private:
double r;
};
#endif
```
cylinder.h:
```cpp
#ifndef CYLINDER_H
#define CYLINDER_H
#include "circle.h"
class Cylinder : public Circle {
public:
Cylinder(double x = 0, double y = 0, double r = 0, double h = 0);
void setH(double h);
double getH() const;
double getVolume() const;
private:
double h;
};
#endif
```
这是成员函数的声明,分别存放在三个源文件中。
point.cpp:
```cpp
#include "point.h"
Point::Point(double x, double y) {
setX(x);
setY(y);
}
void Point::setX(double x) {
this->x = x;
}
double Point::getX() const {
return x;
}
void Point::setY(double y) {
this->y = y;
}
double Point::getY() const {
return y;
}
```
circle.cpp:
```cpp
#include "circle.h"
#include <cmath>
Circle::Circle(double x, double y, double r) : Point(x, y) {
setR(r);
}
void Circle::setR(double r) {
this->r = r;
}
double Circle::getR() const {
return r;
}
double Circle::getArea() const {
return M_PI * r * r;
}
```
cylinder.cpp:
```cpp
#include "cylinder.h"
Cylinder::Cylinder(double x, double y, double r, double h) : Circle(x, y, r) {
setH(h);
}
void Cylinder::setH(double h) {
this->h = h;
}
double Cylinder::getH() const {
return h;
}
double Cylinder::getVolume() const {
return getArea() * h;
}
```
最后,这是成员函数的实现,也分别存放在三个源文件中。
point_impl1.cpp:
```cpp
#include "point.h"
#include <iostream>
using namespace std;
int main() {
Point p(1, 2);
cout << "x = " << p.getX() << ", y = " << p.getY() << endl;
return 0;
}
```
circle_impl1.cpp:
```cpp
#include "circle.h"
#include <iostream>
using namespace std;
int main() {
Circle c(1, 2, 3);
cout << "x = " << c.getX() << ", y = " << c.getY() << ", r = " << c.getR() << endl;
cout << "area = " << c.getArea() << endl;
return 0;
}
```
cylinder_impl1.cpp:
```cpp
#include "cylinder.h"
#include <iostream>
using namespace std;
int main() {
Cylinder c(1, 2, 3, 4);
cout << "x = " << c.getX() << ", y = " << c.getY() << ", r = " << c.getR() << ", h = " << c.getH() << endl;
cout << "volume = " << c.getVolume() << endl;
return 0;
}
```
point_impl2.cpp:
```cpp
#include "point.h"
#include <iostream>
using namespace std;
void printPoint(const Point& p) {
cout << "x = " << p.getX() << ", y = " << p.getY() << endl;
}
int main() {
Point p(1, 2);
printPoint(p);
return 0;
}
```
circle_impl2.cpp:
```cpp
#include "circle.h"
#include <iostream>
using namespace std;
void printCircle(const Circle& c) {
cout << "x = " << c.getX() << ", y = " << c.getY() << ", r = " << c.getR() << endl;
cout << "area = " << c.getArea() << endl;
}
int main() {
Circle c(1, 2, 3);
printCircle(c);
return 0;
}
```
cylinder_impl2.cpp:
```cpp
#include "cylinder.h"
#include <iostream>
using namespace std;
void printCylinder(const Cylinder& c) {
cout << "x = " << c.getX() << ", y = " << c.getY() << ", r = " << c.getR() << ", h = " << c.getH() << endl;
cout << "volume = " << c.getVolume() << endl;
}
int main() {
Cylinder c(1, 2, 3, 4);
printCylinder(c);
return 0;
}
```
point_impl3.cpp:
```cpp
#include "point.h"
Point::Point(double x, double y) {
setX(x);
setY(y);
}
void Point::setX(double x) {
this->x = x;
}
double Point::getX() const {
return x;
}
void Point::setY(double y) {
this->y = y;
}
double Point::getY() const {
return y;
}
```
circle_impl3.cpp:
```cpp
#include "circle.h"
#include <cmath>
Circle::Circle(double x, double y, double r) : Point(x, y) {
setR(r);
}
void Circle::setR(double r) {
this->r = r;
}
double Circle::getR() const {
return r;
}
double Circle::getArea() const {
return M_PI * r * r;
}
```
cylinder_impl3.cpp:
```cpp
#include "cylinder.h"
Cylinder::Cylinder(double x, double y, double r, double h) : Circle(x, y, r) {
setH(h);
}
void Cylinder::setH(double h) {
this->h = h;
}
double Cylinder::getH() const {
return h;
}
double Cylinder::getVolume() const {
return getArea() * h;
}
```
阅读全文
相关推荐
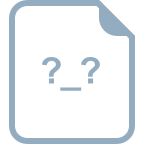














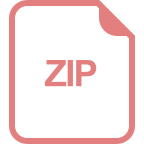