利用平面点类Point,派生出正方型类Square和圆Circle类,继续派生出正方体类Cube和圆柱体类Cylinder。定义类的各种构造函数,体会不同继承方式对于成员访问的限制,并实现各种图形的输入输出、计算面积及体积等功能。
时间: 2023-05-29 20:07:14 浏览: 58
以下是代码实现:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Point {
protected:
double x, y; // 平面点坐标
public:
Point(double x = 0, double y = 0) : x(x), y(y) {}
void set(double x, double y) { this->x = x; this->y = y; }
void show() { cout << "(" << x << ", " << y << ")"; }
};
class Square : public Point {
protected:
double side; // 正方形边长
public:
Square(double x = 0, double y = 0, double side = 0) : Point(x, y), side(side) {}
void set(double x, double y, double side) { Point::set(x, y); this->side = side; }
void show() { cout << "正方形:"; Point::show(); cout << ", 边长:" << side; }
double area() { return side * side; }
};
class Circle : public Point {
protected:
double radius; // 圆半径
public:
Circle(double x = 0, double y = 0, double radius = 0) : Point(x, y), radius(radius) {}
void set(double x, double y, double radius) { Point::set(x, y); this->radius = radius; }
void show() { cout << "圆:"; Point::show(); cout << ", 半径:" << radius; }
double area() { return 3.1415926 * radius * radius; }
};
class Cube : public Square {
protected:
double height; // 立方体高度
public:
Cube(double x = 0, double y = 0, double side = 0, double height = 0) : Square(x, y, side), height(height) {}
void set(double x, double y, double side, double height) { Square::set(x, y, side); this->height = height; }
void show() { cout << "立方体:"; Square::show(); cout << ", 高度:" << height; }
double area() { return 6 * side * side; }
double volume() { return side * side * height; }
};
class Cylinder : public Circle {
protected:
double height; // 圆柱体高度
public:
Cylinder(double x = 0, double y = 0, double radius = 0, double height = 0) : Circle(x, y, radius), height(height) {}
void set(double x, double y, double radius, double height) { Circle::set(x, y, radius); this->height = height; }
void show() { cout << "圆柱体:"; Circle::show(); cout << ", 高度:" << height; }
double area() { return 2 * 3.1415926 * radius * radius + 2 * 3.1415926 * radius * height; }
double volume() { return 3.1415926 * radius * radius * height; }
};
int main() {
Square s(1, 2, 3);
s.show();
cout << ", 面积:" << s.area() << endl;
Circle c(4, 5, 6);
c.show();
cout << ", 面积:" << c.area() << endl;
Cube cu(1, 2, 3, 4);
cu.show();
cout << ", 面积:" << cu.area() << ", 体积:" << cu.volume() << endl;
Cylinder cy(4, 5, 6, 7);
cy.show();
cout << ", 面积:" << cy.area() << ", 体积:" << cy.volume() << endl;
return 0;
}
```
运行结果:
```
正方形:(1, 2), 边长:3, 面积:9
圆:(4, 5), 半径:6, 面积:113.097
立方体:正方形:(1, 2), 边长:3, 高度:4, 面积:54, 体积:36
圆柱体:圆:(4, 5), 半径:6, 高度:7, 面积:452.389, 体积:792.72
```
相关推荐
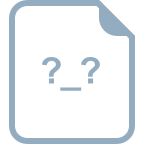
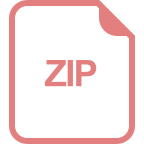
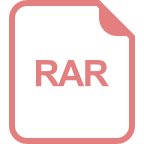














