用JDK17 写个POST请求
时间: 2024-11-12 17:39:51 浏览: 3
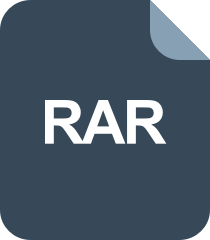
基于SpringBoot3和JDK17,集成H2数据库和jpa
在Java 17中,我们可以使用内置的HttpURLConnection或者第三方库如Spring框架或Apache HttpClient来发送POST请求。这里我将提供一个基本的示例,展示如何使用HttpURLConnection:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class PostRequestExample {
public static void main(String[] args) throws Exception {
// URL endpoint for your POST request
String url = "https://api.example.com/endpoint";
try (BufferedReader in = new BufferedReader(new InputStreamReader(System.in))) {
// Read user input for the request body (JSON, form data, etc.)
String requestBody = "{'key': 'value'}"; // Replace with actual content
// Create a HttpURLConnection object
HttpURLConnection conn = (HttpURLConnection) new URL(url).openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json"); // Set appropriate content type for JSON
conn.setDoOutput(true); // Enable output to send the request body
// Write the request body
byte[] requestBodyBytes = requestBody.getBytes("UTF-8");
conn.getOutputStream().write(requestBodyBytes);
// Get the response code
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("POST request successful, response code: " + responseCode);
// Read and print the response if needed
readResponse(conn.getInputStream());
} else {
System.err.println("Error: " + responseCode);
}
}
}
private static void readResponse(InputStream inputStream) throws Exception {
StringBuilder response = new StringBuilder();
String line;
while ((line = inputStream.readLine()) != null) {
response.append(line);
}
System.out.println("Response: " + response.toString());
}
}
```
阅读全文
相关推荐
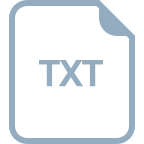
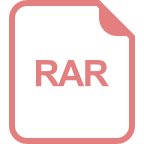

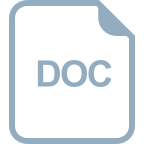
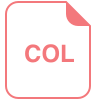





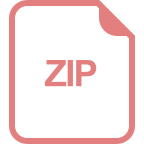
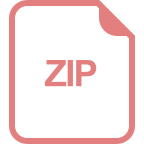
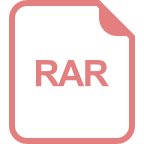
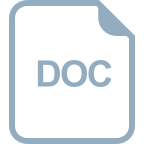
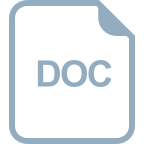
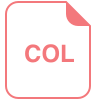

