利用pytorch实现GCN卷积神经网络
时间: 2023-07-31 07:13:57 浏览: 162
GCN(Graph Convolutional Network)是一种用于图数据的卷积神经网络。在PyTorch中,你可以使用DGL(Deep Graph Library)来实现GCN。
首先,确保已经安装了DGL库。你可以使用以下命令来安装:
```
pip install dgl
```
下面是一个简单的示例代码,展示了如何使用DGL来实现GCN:
```python
import dgl
import torch
import torch.nn as nn
import torch.nn.functional as F
from dgl.nn.pytorch import GraphConv
class GCN(nn.Module):
def __init__(self, in_features, hidden_features, out_features):
super(GCN, self).__init__()
self.conv1 = GraphConv(in_features, hidden_features)
self.conv2 = GraphConv(hidden_features, out_features)
def forward(self, g, features):
x = F.relu(self.conv1(g, features))
x = self.conv2(g, x)
return x
# 使用示例
num_nodes = 5 # 图中节点的数量
in_features = 10 # 输入特征的维度
hidden_features = 16 # 隐藏层特征的维度
out_features = 2 # 输出特征的维度
# 创建一个图
g = dgl.graph(([0, 1, 1, 2, 3], [1, 2, 3, 0, 4])) # 定义边的连接方式
g = dgl.add_self_loop(g) # 添加自环
# 创建输入特征张量
features = torch.randn(num_nodes, in_features)
# 创建GCN模型
model = GCN(in_features, hidden_features, out_features)
# 前向传播
output = model(g, features)
print(output)
```
在这个示例中,我们首先使用DGL创建一个图`g`,然后创建一个输入特征张量`features`。接下来,我们定义并创建了一个简单的GCN模型`GCN`,其中使用了`GraphConv`层来实现图卷积操作。最后,我们通过调用模型的`forward`方法来进行前向传播,得到输出结果。
需要注意的是,这只是一个简单的示例,GCN的具体结构和参数设置可以根据具体任务进行调整和改进。另外,DGL还提供了更多的图神经网络模型和操作,你可以根据需要进行进一步的学习和探索。
阅读全文
相关推荐
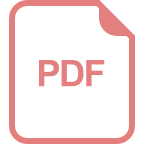
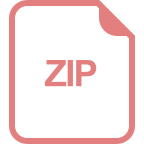

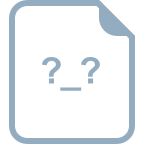
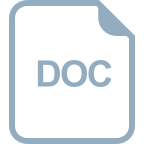
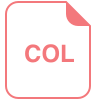


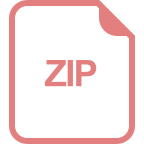
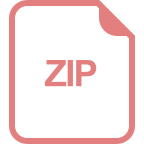
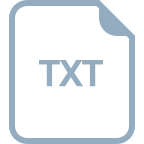
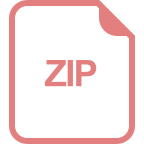
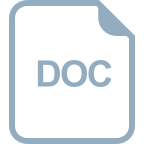
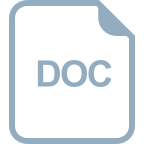
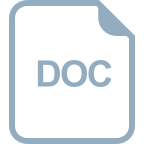
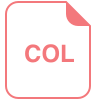
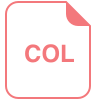
