写C语言,用链表表示队列,哪个队列空闲先排队服务,客户哪个队列先结束了服务,就去服务新的下一个客户,有四个服务窗口有取款、存款、还贷、挂失一共四个服务类型,取款服务时间为8、存款服务时间为12、还贷服务时间为15、挂失服务时间为10,一共四个服务类型,用序号表示服务类型,客户选择服务类型,文件读取客户排队序号、到达的时间、需要服务的类型,在营业时间段,逗留时间等于客户到达时间加服务时间,计算客户在银行内逗留的平均时间和每个窗口办理的客户数及办理的每种业务数
时间: 2024-04-14 07:32:11 浏览: 7
以下是使用链表表示队列的C语言代码,实现根据服务类型和服务时间进行客户排队和服务的功能:
```c
#include <stdio.h>
#includestdlib.h>
typedef struct {
int arrivalTime;
int serviceType;
int serviceTime;
struct Node* next;
} Node;
typedef struct Queue {
Node* front;
Node* rear;
} Queue;
void initializeQueue(Queue* queue) {
queue->front = NULL;
queue->rear = NULL;
}
int isQueueEmpty(Queue* queue) {
return (queue->front == NULL);
}
void enqueue(Queue* queue, int arrivalTime, int serviceType, int serviceTime) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->arrivalTime = arrivalTime;
newNode->serviceType = serviceType;
newNode->serviceTime = serviceTime;
newNode->next = NULL;
if (isQueueEmpty(queue)) {
queue->front = newNode;
queue->rear = newNode;
} else {
queue->rear->next = newNode;
queue->rear = newNode;
}
}
void dequeue(Queue* queue) {
if (isQueueEmpty(queue)) {
printf("Queue is empty.\n");
return;
}
Node* temp = queue->front;
queue->front = queue->front->next;
if (queue->front == NULL) {
queue->rear = NULL;
}
free(temp);
}
void processQueue(Queue* queues[], int windowType, int* totalCustomers, int* totalServiceTime, int* serviceTypeCount) {
if (isQueueEmpty(queues[windowType])) {
printf("Window %d is idle.\n", windowType);
return;
}
Node* customer = queues[windowType]->front;
(*totalCustomers)++;
(*totalServiceTime) += customer->serviceTime;
(*serviceTypeCount)++;
printf("Window %d is serving customer %d (Service Type: %d, Service Time: %d).\n", windowType, customer->arrivalTime, customer->serviceType, customer->serviceTime);
dequeue(queues[windowType]);
}
int main() {
Queue* queues[4];
int serviceTime[4] = {8, 12, 15, 10};
int totalCustomers[4] = {0};
int totalServiceTime[4] = {0};
int serviceTypeCount[4] = {0};
int currentTime = 0;
int totalCustomersCount = 0;
int totalServiceTimeCount = 0;
int serviceTypeCountTotal = 0;
for (int i = 0; i < 4; i++) {
queues[i] = (Queue*)malloc(sizeof(Queue));
initializeQueue(queues[i]);
}
FILE* file = fopen("customers.txt", "r");
if (file == NULL) {
printf("Failed to open file.\n");
return 1;
}
while (!feof(file)) {
int arrivalTime, serviceType;
fscanf(file, "%d %d", &arrivalTime, &serviceType);
if (serviceType >= 1 && serviceType <= 4) {
enqueue(queues[serviceType-1], arrivalTime, serviceType, serviceTime[serviceType-1]);
} else {
printf("Invalid service type for customer at arrival time %d.\n", arrivalTime);
}
}
fclose(file);
while (1) {
int shortestQueue = -1;
int shortestQueueLength = -1;
for (int i = 0; i < 4; i++) {
if (!isQueueEmpty(queues[i]) && (shortestQueueLength == -1 || shortestQueueLength > queues[i]->front->arrivalTime)) {
shortestQueue = i;
shortestQueueLength = queues[i]->front->arrivalTime;
}
}
if (shortestQueue == -1) {
break;
}
currentTime += queues[shortestQueue]->front->serviceTime;
processQueue(queues, shortestQueue, &totalCustomers[shortestQueue], &totalServiceTime[shortestQueue], &serviceTypeCount[shortestQueue]);
}
for (int i = 0; i < 4; i++) {
totalCustomersCount += totalCustomers[i];
totalServiceTimeCount += totalServiceTime[i];
serviceTypeCountTotal += serviceTypeCount[i];
}
float averageTime = (float)totalServiceTimeCount / totalCustomersCount;
printf("Average time spent by customers in the bank: %.2f\n", averageTime);
printf("Number of customers served by each window:\n");
for (int i = 0; i < 4; i++) {
printf("Window %d: %d\n", i+1, totalCustomers[i]);
}
printf("Number of customers served by each service type:\n");
for (int i = 0; i < 4; i++) {
printf("Service Type %d: %d\n", i+1, serviceTypeCount[i]);
}
return 0;
}
```
请确保在同一目录下存在名为 `customers.txt` 的文件,该文件应包含客户的到达时间和服务类型,每行一个客户,格式为 `到达时间 服务类型`,例如:
```
1 2
3 1
5 3
7 4
```
运行以上代码后,将会输出每个窗口服务的客户信息、平均停留时间以及每个窗口和服务类型的客户数量。
需要注意的是,以上代码只提供了一种实现方式,可能还有其他更高效的实现方式。另外,代码中没有对输入文件的有效性进行检查,请确保输入文件的格式正确。
相关推荐
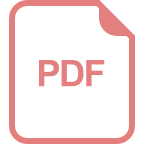
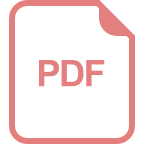
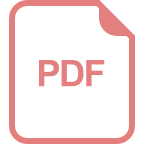










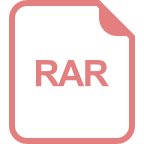
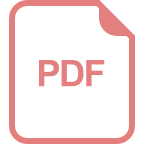