实现linux线程池完成快速排序
时间: 2023-10-14 11:20:03 浏览: 58
好的,我可以为您提供一个简单的实现。这里我将使用C语言和pthread库来实现线程池。
1. 首先,我们需要定义一个结构体来表示线程池中的每个线程。结构体包含线程ID、任务队列、互斥锁和条件变量等。
```
typedef struct {
pthread_t tid; // 线程ID
int index; // 线程池中的索引
int is_working; // 线程是否正在工作
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t cond; // 条件变量
struct task_queue *task_queue; // 任务队列
} thread_pool_t;
```
2. 接着,我们需要定义一个结构体来表示任务。结构体包含任务ID、待排序的数组和数组长度等。
```
typedef struct {
int id; // 任务ID
int *data; // 待排序的数组
int len; // 数组长度
} task_t;
```
3. 我们还需要实现一个任务队列,用于存储待执行的任务。任务队列是一个链表结构,它有一个头指针和一个尾指针,同时也有一个互斥锁用于保护队列的操作。
```
typedef struct task_node {
task_t task;
struct task_node *next;
} task_node_t;
typedef struct task_queue {
task_node_t *head;
task_node_t *tail;
int size;
pthread_mutex_t mutex;
} task_queue_t;
```
4. 接下来,我们需要实现快速排序的算法。这里我们采用经典的递归实现方式。
```
void quick_sort(int *arr, int left, int right) {
if (left >= right) {
return;
}
int i = left;
int j = right;
int pivot = arr[left];
while (i < j) {
while (i < j && arr[j] >= pivot) {
j--;
}
arr[i] = arr[j];
while (i < j && arr[i] <= pivot) {
i++;
}
arr[j] = arr[i];
}
arr[i] = pivot;
quick_sort(arr, left, i - 1);
quick_sort(arr, i + 1, right);
}
```
5. 最后,我们需要编写线程池的主要逻辑。线程池的主要任务是从任务队列中获取任务,并将任务分配给空闲的线程进行处理。当线程池中的所有线程都在工作时,新的任务将被放入任务队列中等待处理。
```
void *thread_func(void *arg) {
thread_pool_t *thread_pool = (thread_pool_t *)arg;
while (1) {
pthread_mutex_lock(&thread_pool->mutex);
while (thread_pool->task_queue->size == 0) {
pthread_cond_wait(&thread_pool->cond, &thread_pool->mutex);
}
task_node_t *task_node = thread_pool->task_queue->head;
thread_pool->task_queue->head = task_node->next;
thread_pool->task_queue->size--;
pthread_mutex_unlock(&thread_pool->mutex);
task_t task = task_node->task;
free(task_node);
thread_pool->is_working = 1;
quick_sort(task.data, 0, task.len - 1);
thread_pool->is_working = 0;
printf("Thread %d finished task %d\n", thread_pool->index, task.id);
}
}
void dispatch_task(thread_pool_t *thread_pool, task_t task) {
task_node_t *task_node = (task_node_t *)malloc(sizeof(task_node_t));
task_node->task = task;
task_node->next = NULL;
pthread_mutex_lock(&thread_pool->mutex);
if (thread_pool->task_queue->size == 0) {
thread_pool->task_queue->head = task_node;
thread_pool->task_queue->tail = task_node;
} else {
thread_pool->task_queue->tail->next = task_node;
thread_pool->task_queue->tail = task_node;
}
thread_pool->task_queue->size++;
pthread_cond_signal(&thread_pool->cond);
pthread_mutex_unlock(&thread_pool->mutex);
}
int main() {
thread_pool_t thread_pool[NUM_THREADS];
task_t tasks[NUM_TASKS];
for (int i = 0; i < NUM_THREADS; i++) {
thread_pool[i].index = i;
thread_pool[i].is_working = 0;
thread_pool[i].task_queue = (task_queue_t *)malloc(sizeof(task_queue_t));
thread_pool[i].task_queue->head = NULL;
thread_pool[i].task_queue->tail = NULL;
thread_pool[i].task_queue->size = 0;
pthread_mutex_init(&thread_pool[i].mutex, NULL);
pthread_cond_init(&thread_pool[i].cond, NULL);
pthread_create(&thread_pool[i].tid, NULL, thread_func, &thread_pool[i]);
}
for (int i = 0; i < NUM_TASKS; i++) {
tasks[i].id = i;
tasks[i].len = ARRAY_LEN;
tasks[i].data = (int *)malloc(sizeof(int) * ARRAY_LEN);
for (int j = 0; j < ARRAY_LEN; j++) {
tasks[i].data[j] = rand() % ARRAY_LEN;
}
dispatch_task(&thread_pool[i % NUM_THREADS], tasks[i]);
}
for (int i = 0; i < NUM_TASKS; i++) {
while (thread_pool[i % NUM_THREADS].is_working);
}
for (int i = 0; i < NUM_THREADS; i++) {
pthread_cancel(thread_pool[i].tid);
pthread_mutex_destroy(&thread_pool[i].mutex);
pthread_cond_destroy(&thread_pool[i].cond);
}
return 0;
}
```
以上代码实现了一个简单的线程池,可以对多个数组进行快速排序。注意,这里我们使用了pthread库的一些线程相关函数,例如pthread_mutex_lock()、pthread_cond_wait()、pthread_create()等。此外,我们还使用了rand()函数来生成随机数组。
相关推荐
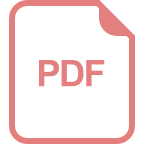
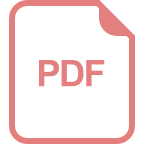
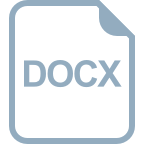














