Linux使用线程池实现快速排序
时间: 2023-12-24 15:46:12 浏览: 70
好的,下面是一个简单的示例代码,使用线程池实现快速排序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_THREADS 100
#define MAX_SIZE 10000
pthread_t threads[MAX_THREADS];
pthread_mutex_t mutex;
int thread_count = 0;
typedef struct {
int* array;
int left;
int right;
} thread_arg;
typedef struct {
thread_arg* args;
int count;
} sort_args;
void* sort_thread(void* arg) {
thread_arg* t_arg = (thread_arg*) arg;
int* array = t_arg->array;
int left = t_arg->left;
int right = t_arg->right;
int pivot = array[right];
int i = left - 1;
for (int j = left; j < right; j++) {
if (array[j] <= pivot) {
i++;
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
int temp = array[i + 1];
array[i + 1] = array[right];
array[right] = temp;
int new_left = left;
int new_right = right;
if (left < i) {
new_left = left;
new_right = i;
thread_arg* new_arg = (thread_arg*) malloc(sizeof(thread_arg));
new_arg->array = array;
new_arg->left = new_left;
new_arg->right = new_right;
pthread_mutex_lock(&mutex);
threads[thread_count++] = 0;
pthread_mutex_unlock(&mutex);
pthread_create(&threads[thread_count - 1], NULL, sort_thread, (void*)new_arg);
}
if (i + 2 < right) {
new_left = i + 2;
new_right = right;
thread_arg* new_arg = (thread_arg*) malloc(sizeof(thread_arg));
new_arg->array = array;
new_arg->left = new_left;
new_arg->right = new_right;
pthread_mutex_lock(&mutex);
threads[thread_count++] = 0;
pthread_mutex_unlock(&mutex);
pthread_create(&threads[thread_count - 1], NULL, sort_thread, (void*)new_arg);
}
pthread_exit(NULL);
}
void quicksort(int* array, int left, int right) {
if (left < right) {
int pivot = array[right];
int i = left - 1;
for (int j = left; j < right; j++) {
if (array[j] <= pivot) {
i++;
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
int temp = array[i + 1];
array[i + 1] = array[right];
array[right] = temp;
if (thread_count < MAX_THREADS) {
int new_left = left;
int new_right = right;
if (left < i) {
new_left = left;
new_right = i;
thread_arg* new_arg = (thread_arg*) malloc(sizeof(thread_arg));
new_arg->array = array;
new_arg->left = new_left;
new_arg->right = new_right;
pthread_mutex_lock(&mutex);
threads[thread_count++] = 0;
pthread_mutex_unlock(&mutex);
pthread_create(&threads[thread_count - 1], NULL, sort_thread, (void*)new_arg);
}
if (i + 2 < right) {
new_left = i + 2;
new_right = right;
thread_arg* new_arg = (thread_arg*) malloc(sizeof(thread_arg));
new_arg->array = array;
new_arg->left = new_left;
new_arg->right = new_right;
pthread_mutex_lock(&mutex);
threads[thread_count++] = 0;
pthread_mutex_unlock(&mutex);
pthread_create(&threads[thread_count - 1], NULL, sort_thread, (void*)new_arg);
}
} else {
quicksort(array, left, i);
quicksort(array, i + 2, right);
}
}
}
void parallel_quicksort(int* array, int left, int right) {
thread_count = 0;
pthread_mutex_init(&mutex, NULL);
thread_arg* arg = (thread_arg*) malloc(sizeof(thread_arg));
arg->array = array;
arg->left = left;
arg->right = right;
threads[thread_count++] = 0;
pthread_create(&threads[thread_count - 1], NULL, sort_thread, (void*)arg);
for (int i = 0; i < thread_count; i++) {
pthread_join(threads[i], NULL);
}
pthread_mutex_destroy(&mutex);
}
int main() {
int array[MAX_SIZE];
int n;
printf("Enter the number of elements: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("Enter element %d: ", i + 1);
scanf("%d", &array[i]);
}
parallel_quicksort(array, 0, n - 1);
printf("Sorted array: ");
for (int i = 0; i < n; i++) {
printf("%d ", array[i]);
}
printf("\n");
return 0;
}
```
该示例代码使用了线程池来实现多线程快速排序。当线程池中的线程数量达到最大值时,程序将使用递归方式继续排序。在 `parallel_quicksort` 函数中,程序初始化了一个互斥锁(`mutex`),然后创建一个线程来执行快速排序。在 `sort_thread` 函数中,线程完成了数组的排序,并根据情况创建新的线程来继续排序。在整个程序执行期间,主线程会等待所有线程都执行完毕后再退出。
阅读全文
相关推荐







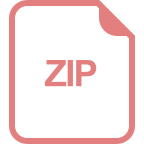
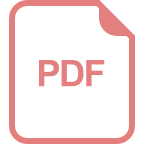
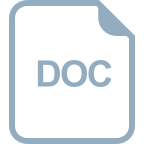
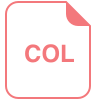
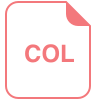
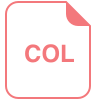
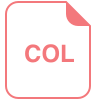
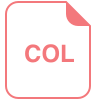
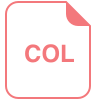