(1)顺序栈的进、出栈,链栈的进、出栈; (2)顺序队列的入队、出队,链队列的入队、出队。的例子加上运行后的代码图
时间: 2024-10-23 18:08:46 浏览: 19
(1)顺序栈(数组实现):
- 进栈:`push()`操作,将元素添加到栈顶。例如,在Python中,可以这样做:
```python
def push(s, x):
s.append(x)
```
- 出栈:`pop()`操作,移除并返回栈顶元素。如果栈为空,则会抛出异常。示例:
```python
def pop(s):
if len(s) == 0:
raise Exception("Stack is empty")
return s.pop()
```
链栈(链表实现):
- 进栈:创建一个新的节点并将其链接到栈顶。如C++中:
```cpp
void push(Stack * &s, int val) {
StackNode* newNode = new StackNode(val);
newNode->next = s->top;
s->top = newNode;
}
```
- 出栈:删除并返回栈顶元素。若栈空则不操作。C++示例:
```cpp
int pop(Stack * &s) {
if (s->top == NULL) return -1;
int topVal = s->top->val;
StackNode* temp = s->top;
s->top = s->top->next;
delete temp;
return topVal;
}
```
(2)顺序队列(数组实现):
- 入队:`enqueue()`,在队尾添加元素。Python示例:
```python
def enqueue(q, x):
q.append(x)
```
- 出队:`dequeue()`,从队头移除并返回元素。若队列为空则报错。Python示例:
```python
def dequeue(q):
if len(q) == 0:
raise Exception("Queue is empty")
return q.pop(0)
```
链队列(链表实现):
- 入队:创建新节点并将它连接到队尾。C++示例:
```cpp
void enqueue(Queue * &q, int val) {
Node* newNode = new Node(val);
newNode->next = q->tail;
q->tail = newNode;
if (!q->head) q->head = newNode;
}
```
- 出队:删除并返回队头元素。如果队列为空则返回null。C++示例:
```cpp
int dequeue(Queue * &q) {
if (q->head == NULL) return -1;
int frontVal = q->head->val;
Node* temp = q->head;
q->head = q->head->next;
delete temp;
return frontVal;
}
```
以上代码并未绘制实际的运行时图,因为这是文本描述,但在实际开发环境中,你可以通过图形界面或者打印链表结构来可视化这些操作的过程。
阅读全文
相关推荐
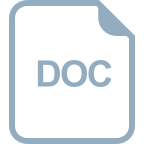
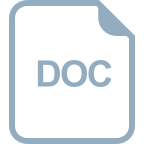
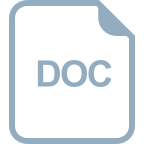
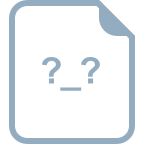
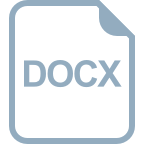
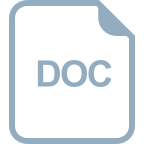
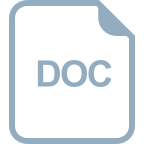
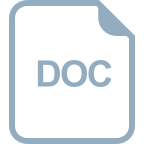
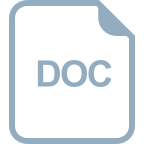
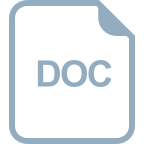
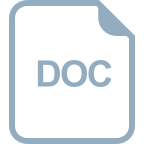
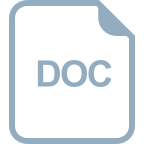
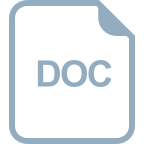
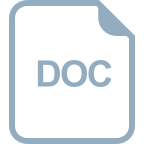
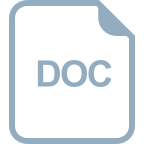
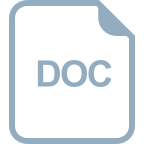


