分析完成def fit_naive_bayes_model(matrix, labels): """Fit a naive bayes model. This function should fit a Naive Bayes model given a training matrix and labels. The function should return the state of that model. Feel free to use whatever datatype you wish for the state of the model. Args: matrix: A numpy array containing word counts for the training data labels: The binary (0 or 1) labels for that training data Returns: The trained model """ # *** START CODE HERE *** # *** END CODE HERE ***
时间: 2023-11-22 16:52:29 浏览: 37
这是一个用于训练朴素贝叶斯模型的函数。输入参数是一个numpy数组类型的训练矩阵和标签向量。其中,训练矩阵中包含了单词的计数信息,标签向量则对应每个样本的二进制标签。该函数需要返回训练后的朴素贝叶斯模型。
具体实现思路如下:
1. 定义计算先验概率函数P(Y)的函数prior_probability(labels)。
2. 定义计算条件概率函数P(X|Y)的函数conditional_probability(matrix, labels)。
3. 计算先验概率和条件概率,并将它们存储在一个字典类型的模型state中。
4. 返回模型state。
具体实现代码如下:
```python
import numpy as np
def fit_naive_bayes_model(matrix, labels):
"""
Fit a naive bayes model.
This function should fit a Naive Bayes model given a training matrix and labels.
The function should return the state of that model.
Feel free to use whatever datatype you wish for the state of the model.
Args:
matrix: A numpy array containing word counts for the training data
labels: The binary (0 or 1) labels for that training data
Returns: The trained model
"""
num_docs, num_words = matrix.shape
state = {}
# Compute prior probabilities
state['prior'] = prior_probability(labels)
# Compute conditional probabilities
state['conditional'] = conditional_probability(matrix, labels)
return state
def prior_probability(labels):
num_docs = len(labels)
num_spam = np.sum(labels)
p_spam = num_spam / num_docs
p_not_spam = 1 - p_spam
return {'spam': p_spam, 'not_spam': p_not_spam}
def conditional_probability(matrix, labels):
num_docs, num_words = matrix.shape
num_spam = np.sum(labels)
num_not_spam = num_docs - num_spam
spam_matrix = matrix[labels == 1]
not_spam_matrix = matrix[labels == 0]
spam_word_counts = np.sum(spam_matrix, axis=0)
not_spam_word_counts = np.sum(not_spam_matrix, axis=0)
spam_total_words = np.sum(spam_word_counts)
not_spam_total_words = np.sum(not_spam_word_counts)
spam_probs = (spam_word_counts + 1) / (spam_total_words + num_words)
not_spam_probs = (not_spam_word_counts + 1) / (not_spam_total_words + num_words)
return {'spam': spam_probs, 'not_spam': not_spam_probs}
```
其中,prior_probability函数用于计算先验概率,conditional_probability函数用于计算条件概率。这里的条件概率指的是在给定类别的情况下,每个单词出现的概率。在计算条件概率时,为了避免概率值为0,这里采用了拉普拉斯平滑的方法。最后,将先验概率和条件概率存储在一个字典类型的state中,并返回该模型。
相关推荐
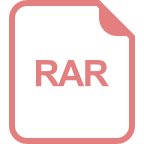
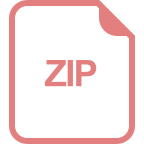














