linux msgget使用
时间: 2023-05-24 13:06:16 浏览: 186
msgget是Linux进程间通信(IPC)中的一个函数,用于创建或者打开一个消息队列。具体使用方法如下:
```c++
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
int msgget(key_t key, int msgflg);
```
参数说明:
- key:消息队列关键字,一个整数值,不同的值代表不同的消息队列。
- msgflg:flags参数,表示消息队列的操作模式,取值可以是以下几种模式的或者:
| 模式标志 | 描述 |
| :------- | :-------------------------- |
| IPC_CREAT | 如果没有此消息队列则创建一个,如果已存在则返回该消息队列的ID |
| IPC_EXCL | 与IPC_CREAT一起使用,若该消息队列已存在,则返回错误码 |
| IPC_PRIVATE | 该标志唯一的用途就是与IPC_RMID一起使用 |
返回值:
- 若调用成功,则返回一个非负整数——消息队列的ID。
- 若出现错误,则返回-1,并设置errno为相应值。
示例代码:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_MAX_SIZE 1024
struct msgbuf
{
long mtype;
char mtext[MSG_MAX_SIZE];
};
int main(int argc, char **argv)
{
int msgid = 0;
key_t key = 123456;
struct msgbuf msg;
memset(&msg, 0x00, sizeof(struct msgbuf));
// 通过key创建或打开一个消息队列
msgid = msgget(key, IPC_CREAT);
if (msgid == -1)
{
printf("%d:%s\n", errno, strerror(errno));
exit(EXIT_FAILURE);
}
printf("create msg queue :%d\n", msgid);
// 发送消息到消息队列
msg.mtype = 1;
strcpy(msg.mtext, "hello, this is a message!");
if (msgsnd(msgid, (void *) &msg, MSG_MAX_SIZE, IPC_NOWAIT) == -1)
{
printf("%d:%s\n", errno, strerror(errno));
exit(EXIT_FAILURE);
}
printf("send message success\n");
return 0;
}
```
在这个示例程序中,我们定义了一个`struct msgbuf`结构体用来存放消息,其中`long mtype`是消息类型,`char mtext[MSG_MAX_SIZE]`是消息文本内容。在程序中,我们通过`msgget`函数创建或打开一个消息队列,然后利用`msgsnd`函数向消息队列中发送一条消息。
需要注意的是,msgget成功调用后,我们最好存储一下返回的msgid,以便于后面在程序中使用。而在Linux下,使用IPC通讯时,需要定义key值,用于标识IPC通讯中的对象,这个key可以是手动指定的,比如这个示例程序中的123456,也可以使用ftok函数从指定的文件路径中生成。唯一的要求是,所有使用IPC通讯的进程使用的key值必须是一样的。
阅读全文
相关推荐
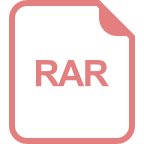
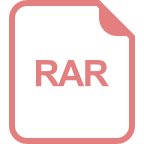
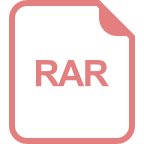

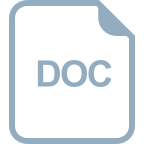

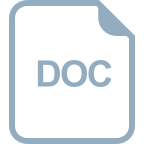
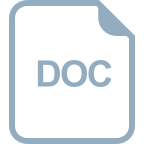
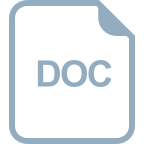









