ga-svm多分类python
时间: 2023-09-08 22:09:39 浏览: 97
遗传算法(Genetic Algorithm,GA)是一种基于生物进化过程中的基因遗传、交叉和变异等机制,通过不断迭代寻找最优解的优化算法。在SVM多分类问题中,GA可以用来优化SVM中的参数,以达到更好的分类效果。
下面是一个使用遗传算法优化SVM多分类的Python示例:
1. 准备数据集:同样需要准备一个用于训练SVM多分类模型的数据集。
2. 定义SVM模型:同样使用sklearn库中的SVM模型来进行多分类训练。
3. 定义GA算法的适应度函数:在SVM中,适应度函数可以定义为分类准确率,即被正确分类的样本数占总样本数的比例。
4. 定义GA算法的染色体编码:可以使用二进制编码来表示SVM模型中的参数,如对于SVM中的C和gamma参数,可以将其分别编码为二进制串。
5. 实现GA算法:使用Python实现GA算法,按照上述步骤进行迭代,直到找到最优解或到达最大迭代次数。
6. 测试模型:使用测试集对训练好的模型进行测试,计算出模型的准确率和其他评价指标。
需要注意的是,GA算法的收敛速度相对较慢,适合用于解决复杂的优化问题。在SVM多分类问题中,可以使用交叉验证等技术来提高模型的训练效果。
相关问题
ga-svm多分类python代码
以下是一个使用遗传算法优化SVM多分类的Python示例代码:
```python
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
from sklearn.svm import SVC
import numpy as np
import random
# 准备数据集
X, y = load_data() # 加载数据集
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 定义SVM模型
clf = SVC()
# 定义GA算法的适应度函数
def fitness(population):
accuracies = []
for chromosome in population:
clf.set_params(C=chromosome[0], gamma=chromosome[1])
clf.fit(X_train, y_train)
y_pred = clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
accuracies.append(accuracy)
return accuracies
# 定义GA算法的染色体编码
def chromosome_encoding(population_size):
population = []
for i in range(population_size):
chromosome = [random.uniform(0.1, 10), random.uniform(0.001, 1)]
population.append(chromosome)
return population
# 定义GA算法的交叉操作
def crossover(parent1, parent2):
child1 = [parent1[0], parent2[1]]
child2 = [parent2[0], parent1[1]]
return child1, child2
# 定义GA算法的变异操作
def mutation(chromosome):
mutation_prob = 0.1
if random.random() < mutation_prob:
chromosome[random.randint(0, 1)] = random.uniform(0.1, 10)
return chromosome
# 实现GA算法
population_size = 50
num_generations = 100
population = chromosome_encoding(population_size)
for i in range(num_generations):
fitness_values = fitness(population)
sorted_indices = np.argsort(fitness_values)[::-1]
population = [population[i] for i in sorted_indices]
next_generation = [population[0]]
for j in range(1, population_size):
parent1 = population[random.randint(0, population_size // 2)]
parent2 = population[random.randint(0, population_size // 2)]
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1)
child2 = mutation(child2)
next_generation.extend([child1, child2])
population = next_generation[:population_size]
# 测试模型
best_chromosome = population[0]
clf.set_params(C=best_chromosome[0], gamma=best_chromosome[1])
clf.fit(X_train, y_train)
y_pred = clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy: {:.2f}%".format(accuracy * 100))
```
在上述代码中,我们首先加载数据集并划分训练集和测试集。然后定义SVM模型、适应度函数、染色体编码、交叉操作和变异操作。接着使用遗传算法进行训练,并测试模型的准确率。最后输出模型的准确率。需要注意的是,在实际应用中,可以进行交叉验证等技术来提高模型的泛化能力。
ga-svm的python代码
ga-svm是一种支持向量机(Support Vector Machine)算法的变种,它使用遗传算法(Genetic Algorithm)来优化支持向量机的超参数。
下面是一个基于Python的实现代码示例:
```python
import numpy as np
from sklearn.svm import SVC
from sklearn.model_selection import cross_val_score
# 设置遗传算法参数
POPULATION_SIZE = 50 # 种群大小
GENERATION_NUM = 100 # 迭代代数
CROSSOVER_PROB = 0.8 # 交叉概率
MUTATION_PROB = 0.1 # 变异概率
# 初始化种群
def init_population():
population = []
for _ in range(POPULATION_SIZE):
# 随机生成C和gamma的取值
C = np.random.uniform(0.1, 10)
gamma = np.random.uniform(0.1, 5)
population.append([C, gamma])
return population
# 评估适应度函数
def evaluate_fitness(population, X, y):
fitness = []
for ind in population:
# 创建支持向量机模型,使用交叉验证计算适应度
svc = SVC(C=ind[0], gamma=ind[1])
score = cross_val_score(svc, X, y, cv=5).mean() # 5折交叉验证
fitness.append(score)
return fitness
# 选择操作
def selection(population, fitness):
# 根据适应度值进行排序
sorted_indices = np.argsort(fitness)
# 选择适应度较高的个体
selected_population = [population[i] for i in sorted_indices[-POPULATION_SIZE:]]
return selected_population
# 交叉操作
def crossover(population):
new_population = []
for _ in range(POPULATION_SIZE):
# 随机选择两个个体
parent1, parent2 = np.random.choice(population, size=2, replace=False)
if np.random.rand() < CROSSOVER_PROB:
# 按一定比例交叉生成新个体
child = [parent1[0], parent2[1]]
else:
# 保留原个体
child = parent1
new_population.append(child)
return new_population
# 变异操作
def mutation(population):
for ind in population:
if np.random.rand() < MUTATION_PROB:
# 对C和gamma进行随机变异
ind[0] = np.random.uniform(0.1, 10)
ind[1] = np.random.uniform(0.1, 5)
return population
# 主函数
def ga_svm(X, y):
population = init_population()
for _ in range(GENERATION_NUM):
fitness = evaluate_fitness(population, X, y)
population = selection(population, fitness)
population = crossover(population)
population = mutation(population)
# 选择最佳个体
best_ind = population[np.argmax(fitness)]
return best_ind
# 使用示例
X = np.array([[0, 0], [1, 1]])
y = np.array([0, 1])
best_ind = ga_svm(X, y)
print('Best individual:', best_ind)
```
以上是一个用Python实现的基于遗传算法的SVM代码示例,该代码通过遗传算法优化SVM的超参数选取,以获得更好的分类性能。代码中使用了`sklearn`库中的SVC类来建立支持向量机模型,使用5折交叉验证评估模型性能。遗传算法中的选择、交叉和变异操作通过相应的函数实现。最终输出的是训练出的最佳个体,对应的C和gamma参数。这个示例可以根据具体的数据集和问题进行配置和调整。
相关推荐
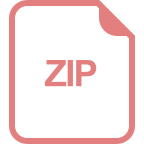
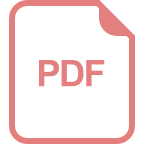












