GA优化SVMpython代码
时间: 2023-10-13 12:21:16 浏览: 53
以下是使用遗传算法优化SVM超参数的Python代码示例:
```python
from sklearn.datasets import load_iris
from sklearn.svm import SVC
from sklearn.model_selection import cross_val_score
from deap import base, creator, tools, algorithms
import numpy as np
# 加载数据集
iris = load_iris()
X = iris.data
y = iris.target
# 定义适应度函数
def evaluate(individual):
# 提取超参数
C, gamma = individual
# 训练SVM模型并计算交叉验证分数
svm = SVC(C=C, gamma=gamma, random_state=42)
scores = cross_val_score(svm, X, y, cv=5)
# 返回平均交叉验证分数作为适应度
return np.mean(scores),
# 定义遗传算法参数
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
toolbox = base.Toolbox()
toolbox.register("attr_float", np.random.uniform, 0, 10)
toolbox.register("individual", tools.initCycle, creator.Individual,
(toolbox.attr_float,)*2, n=1)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("evaluate", evaluate)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutFlipBit, indpb=0.05)
toolbox.register("select", tools.selTournament, tournsize=3)
# 运行遗传算法
population = toolbox.population(n=10)
for gen in range(10):
offspring = algorithms.varAnd(population, toolbox, cxpb=0.5, mutpb=0.1)
fits = toolbox.map(toolbox.evaluate, offspring)
for fit, ind in zip(fits, offspring):
ind.fitness.values = fit
population = toolbox.select(offspring, k=len(population))
top1 = tools.selBest(population, k=1)[0]
print("Generation {}, Best Fitness: {}".format(gen+1, top1.fitness.values[0]))
print("Best Individual: ", top1)
# 输出最佳超参数和分数
best_ind = tools.selBest(population, k=1)[0]
best_params = best_ind[0], best_ind[1]
svm = SVC(C=best_params[0], gamma=best_params[1], random_state=42)
scores = cross_val_score(svm, X, y, cv=5)
print("Best C: {}, Best Gamma: {}".format(best_params[0], best_params[1]))
print("Best Cross Validation Score: {:.2f}".format(np.mean(scores)))
```
上述代码使用DEAP库实现了遗传算法的各个组件,并将其应用于SVM超参数优化。其中,适应度函数使用5折交叉验证来评估SVM模型的性能。遗传算法的主要参数包括种群大小、交叉概率、变异概率等。运行遗传算法后,输出最佳超参数和分数。
相关推荐
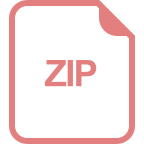













