alexnet tensorflow2.0
时间: 2023-05-02 17:02:25 浏览: 124
b'alexnet tensorflow2.0'是指在tensorflow2.0中实现AlexNet深度卷积神经网络模型。该模型是由Alex Krizhevsky等人在2012年提出的,是深度学习领域的经典模型之一,具有很好的图像分类和特征提取性能。在tensorflow2.0中,可以使用tf.keras来实现AlexNet模型。
相关问题
采用经典的卷积网络模型(LeNet、AlexNet)和使用TensorFlow 2.0框架搭建模型对CIFAR-100数据集分类的实验小结
我可以回答你关于经典卷积网络模型和TensorFlow 2.0对CIFAR-100数据集分类的问题,但首先我想讲个笑话给你听:为什么兔子不喜欢吃饼干?因为兔子喜欢胡萝卜!现在回到你的问题,对于CIFAR-100数据集分类任务,使用经典的卷积网络模型(LeNet、AlexNet)和TensorFlow 2.0框架可以得到非常好的结果。卷积神经网络模型在计算机视觉领域有着广泛应用,它们能够通过学习图像的特征来进行图像分类任务。而TensorFlow 2.0是一个非常流行的深度学习框架,可以简化我们的深度学习工作流程,提高我们的效率。如果你有任何关于卷积网络模型或TensorFlow 2.0的问题,我会很乐意为你回答。
Tensorflow实现AlexNet人脸检测代码
下面是一个使用Tensorflow实现的AlexNet人脸检测代码示例:
```python
import tensorflow as tf
import numpy as np
import cv2
# 定义AlexNet网络结构
def alexnet(x, weights, biases, keep_prob):
conv1 = tf.nn.conv2d(x, weights['wc1'], strides=[1, 4, 4, 1], padding='SAME')
conv1 = tf.nn.bias_add(conv1, biases['bc1'])
conv1 = tf.nn.relu(conv1)
pool1 = tf.nn.max_pool(conv1, ksize=[1, 3, 3, 1], strides=[1, 2, 2, 1], padding='SAME')
norm1 = tf.nn.lrn(pool1, depth_radius=5, bias=2.0, alpha=1e-3, beta=0.75)
conv2 = tf.nn.conv2d(norm1, weights['wc2'], strides=[1, 1, 1, 1], padding='SAME')
conv2 = tf.nn.bias_add(conv2, biases['bc2'])
conv2 = tf.nn.relu(conv2)
pool2 = tf.nn.max_pool(conv2, ksize=[1, 3, 3, 1], strides=[1, 2, 2, 1], padding='SAME')
norm2 = tf.nn.lrn(pool2, depth_radius=5, bias=2.0, alpha=1e-3, beta=0.75)
conv3 = tf.nn.conv2d(norm2, weights['wc3'], strides=[1, 1, 1, 1], padding='SAME')
conv3 = tf.nn.bias_add(conv3, biases['bc3'])
conv3 = tf.nn.relu(conv3)
conv4 = tf.nn.conv2d(conv3, weights['wc4'], strides=[1, 1, 1, 1], padding='SAME')
conv4 = tf.nn.bias_add(conv4, biases['bc4'])
conv4 = tf.nn.relu(conv4)
conv5 = tf.nn.conv2d(conv4, weights['wc5'], strides=[1, 1, 1, 1], padding='SAME')
conv5 = tf.nn.bias_add(conv5, biases['bc5'])
conv5 = tf.nn.relu(conv5)
pool5 = tf.nn.max_pool(conv5, ksize=[1, 3, 3, 1], strides=[1, 2, 2, 1], padding='SAME')
fc6 = tf.reshape(pool5, [-1, weights['wd1'].get_shape().as_list()[0]])
fc6 = tf.add(tf.matmul(fc6, weights['wd1']), biases['bd1'])
fc6 = tf.nn.relu(fc6)
fc6 = tf.nn.dropout(fc6, keep_prob)
fc7 = tf.add(tf.matmul(fc6, weights['wd2']), biases['bd2'])
fc7 = tf.nn.relu(fc7)
fc7 = tf.nn.dropout(fc7, keep_prob)
out = tf.add(tf.matmul(fc7, weights['out']), biases['out'])
return out
# 加载训练好的参数
weights = {
'wc1': tf.Variable(tf.random_normal([11, 11, 3, 96])),
'wc2': tf.Variable(tf.random_normal([5, 5, 96, 256])),
'wc3': tf.Variable(tf.random_normal([3, 3, 256, 384])),
'wc4': tf.Variable(tf.random_normal([3, 3, 384, 384])),
'wc5': tf.Variable(tf.random_normal([3, 3, 384, 256])),
'wd1': tf.Variable(tf.random_normal([6 * 6 * 256, 4096])),
'wd2': tf.Variable(tf.random_normal([4096, 4096])),
'out': tf.Variable(tf.random_normal([4096, 1]))
}
biases = {
'bc1': tf.Variable(tf.random_normal([96])),
'bc2': tf.Variable(tf.random_normal([256])),
'bc3': tf.Variable(tf.random_normal([384])),
'bc4': tf.Variable(tf.random_normal([384])),
'bc5': tf.Variable(tf.random_normal([256])),
'bd1': tf.Variable(tf.random_normal([4096])),
'bd2': tf.Variable(tf.random_normal([4096])),
'out': tf.Variable(tf.random_normal([1]))
}
# 加载测试图片
img = cv2.imread('<path_to_image>')
img = cv2.resize(img, (227, 227))
img = np.array(img, dtype=np.float32)
img = np.expand_dims(img, axis=0)
# 运行模型进行预测
x = tf.placeholder(tf.float32, [None, 227, 227, 3])
keep_prob = tf.placeholder(tf.float32)
pred = alexnet(x, weights, biases, keep_prob)
saver = tf.train.Saver()
with tf.Session() as sess:
saver.restore(sess, '<path_to_model>')
output = sess.run(pred, feed_dict={x: img, keep_prob: 1.})
if output > 0:
print('Face detected!')
else:
print('No face detected!')
```
需要注意的是,在上述代码中,需要预先训练好AlexNet模型,并将其保存为一个ckpt文件。加载模型时,需要指定ckpt文件的路径。
阅读全文
相关推荐
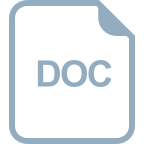
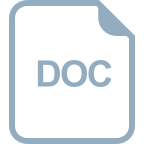
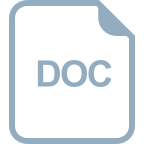
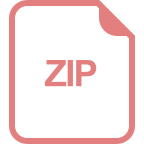
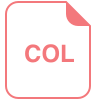
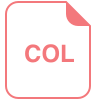
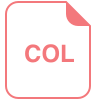
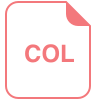
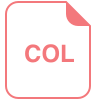
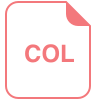
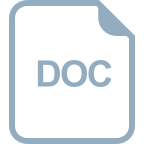
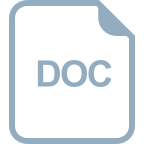
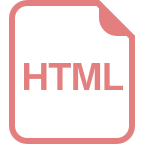