torch::from_blob用法
时间: 2023-10-09 09:13:50 浏览: 105
`torch::from_blob` 是 PyTorch C++ API 中用于将一段内存转换为 `torch::Tensor` 的函数。
它的使用方法如下:
```c++
#include <torch/torch.h>
int main() {
float data[] = {1, 2, 3, 4, 5, 6};
auto tensor = torch::from_blob(data, {2, 3});
std::cout << tensor << std::endl;
return 0;
}
```
`from_blob` 函数接收两个参数:
- `data`:需要转换为 `torch::Tensor` 的内存地址。
- `sizes`:一个整数 vector,表示该内存块对应的 tensor 的形状。
注意:
- `from_blob` 函数不会拷贝数据,而是将数据指针包装成 tensor。因此,如果内存块在 tensor 生命周期内被释放,tensor 将会变得无效。
- `from_blob` 函数默认将数据按照 C 语言风格的连续内存块存储,即按照行优先的顺序存储。如果内存块的存储方式不同,需要使用 `torch::TensorOptions` 重新指定存储方式。
- `from_blob` 函数默认将数据类型解释为 float,如果需要指定其他数据类型,可以使用 `torch::TensorOptions` 指定数据类型。
相关问题
VS2019使用加载的 TorchScript 模型进行图像推断
可以使用 PyTorch 的 C++ 绑定来在 C++ 中使用加载的 TorchScript 模型进行图像推断。
首先,需要在 Visual Studio 中创建一个 C++ 项目,并在项目中安装 PyTorch 的 C++ 绑定。
然后,需要使用 torch::jit::load() 函数加载 TorchScript 模型。这个函数接受一个文件路径作为参数,并返回一个 torch::jit::script::Module 类型的对象,表示加载的模型。
接下来,可以使用这个模型对象的 forward() 方法对图像进行推断。这个方法接受一个 torch::Tensor 类型的输入,并返回一个 torch::Tensor 类型的输出。
例如,以下是一个简单的示例代码,展示了如何使用加载的 TorchScript 模型对图像进行推断:
```
#include <torch/script.h>
#include <opencv2/opencv.hpp>
int main() {
// 加载模型
auto model = torch::jit::load("model.pt");
// 读入图像
cv::Mat image = cv::imread("image.jpg");
// 将图像转换为 PyTorch Tensor
torch::Tensor tensor_image = torch::from_blob(image.data, {1, image.rows, image.cols, 3}, torch::kByte);
tensor_image = tensor_image.permute({0, 3, 1, 2});
tensor_image = tensor_image.to(torch::kF32);
// 使用模型进行推断
std::vector<torch::jit::IValue> inputs = {tensor_image};
auto output = model.forward(inputs).toTensor();
// 处理输出
// ...
}
```
在这个例子中,我们使用 Open
onnxruntime tensor格式转成torch格式 c++版本
将ONNXRuntime的Tensor转换为PyTorch的Tensor格式可以使用以下C++代码:
```c++
#include <onnxruntime_cxx_api.h>
#include <torch/torch.h>
// 加载ONNX模型和ONNXRuntime的执行提供程序
Ort::SessionOptions options;
Ort::Env env(ORT_LOGGING_LEVEL_WARNING, "test");
Ort::Session session(env, "model.onnx", options);
Ort::AllocatorWithDefaultOptions allocator;
// 构造输入数据
std::vector<int64_t> input_shape = {batch_size, channels, height, width};
std::vector<float> input_data(batch_size * channels * height * width);
std::generate(input_data.begin(), input_data.end(), std::rand);
// 创建ONNXRuntime的输入Tensor
Ort::MemoryInfo memory_info = Ort::MemoryInfo::CreateCpu(OrtDeviceAllocator, OrtMemTypeDefault);
Ort::Value input_tensor = Ort::Value::CreateTensor<float>(memory_info, input_data.data(), input_data.size(), input_shape.data(), input_shape.size());
// 将ONNXRuntime的Tensor转换为numpy数组
auto output_tensors = session.Run(Ort::RunOptions{nullptr}, input_names.data(), &input_tensor, 1, output_names.data(), output_names.size());
auto output_tensor = output_tensors.front().Get<Tensor>();
auto output_shape = output_tensor.Shape().GetDims();
auto output_data = output_tensor.Data<float>();
// 将numpy数组转换为PyTorch的Tensor
auto output = torch::from_blob(output_data, output_shape).clone();
```
其中,`model.onnx`是ONNX格式的模型文件,`batch_size`、`channels`、`height`、`width`是输入数据的形状。`input_data`是输入数据的数组,`output_data`是转换后的输出数据的数组,`output_shape`是输出数据的形状。`output`是转换后的PyTorch的Tensor。值得注意的是,在将ONNXRuntime的Tensor转换为numpy数组时,需要先通过`session.Run()`方法获取输出张量,然后再通过`output_tensor.Data<float>()`获取输出张量的数据。
相关推荐
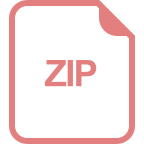
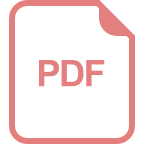










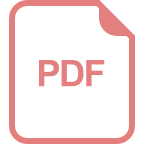
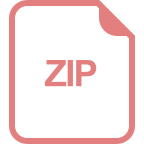