C语言实现cuckoo hash
时间: 2023-02-23 22:51:19 浏览: 232
Cuckoo Hashing是一种Hash表的实现方式,它的优点在于具有高效率、少冲突和快速查找的特点。C语言可以通过定义一个结构体来存储元素,并使用指针进行链接,然后使用哈希函数将元素映射到表中的桶中。下面是一个C语言实现Cuckoo Hashing的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 10
struct Node {
int key;
int value;
};
struct HashTable {
struct Node *table[SIZE];
};
int hash1(int key) {
return key % SIZE;
}
int hash2(int key) {
return (key / SIZE) % SIZE;
}
void insert(struct HashTable *ht, int key, int value) {
int h1 = hash1(key);
int h2 = hash2(key);
struct Node *n = (struct Node*) malloc(sizeof(struct Node));
n->key = key;
n->value = value;
ht->table[h1] = n;
ht->table[h2] = n;
}
int main() {
struct HashTable ht;
memset(ht.table, 0, sizeof(ht.table));
insert(&ht, 1, 10);
insert(&ht, 2, 20);
insert(&ht, 3, 30);
printf("%d\n", ht.table[hash1(1)]->value);
printf("%d\n", ht.table[hash2(2)]->value);
printf("%d\n", ht.table[hash1(3)]->value);
return 0;
}
```
这仅仅是一个简单的示例,实际上,Cuckoo Hashing还需要实现许多其他功能,例如判断哈希冲突、进行重构等。但是,上面的代码可以作为C语言实现Cuckoo Hashing的一个基础。
阅读全文
相关推荐

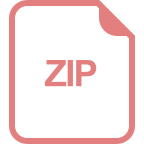
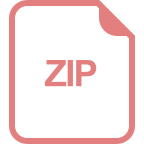


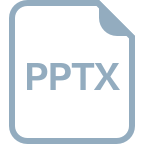
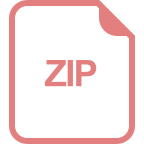
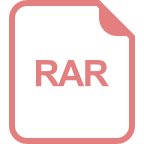
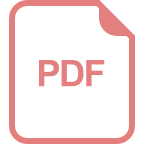
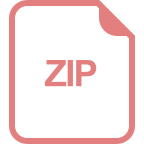
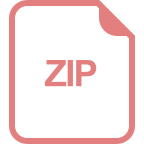
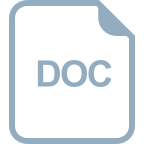
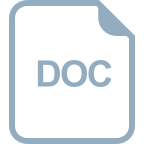
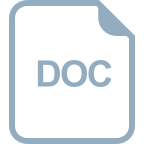

