cuckoo hash代码
时间: 2023-08-31 08:20:39 浏览: 29
### 回答1:
下面是一个简单的C++实现cuckoo hash的代码,其中包含了插入、查找和删除操作:
```c++
#include <iostream>
#include <vector>
using namespace std;
const int MAXN = 100007;
const int hash_num = 2; // 哈希函数个数
const int max_loop = 500; // 最大循环次数
int n, m;
vector<int> h[hash_num][MAXN]; // 存储哈希表
// 哈希函数1
int hash1(int x) {
return x % MAXN;
}
// 哈希函数2
int hash2(int x) {
return (x / MAXN) % MAXN;
}
// 插入元素
void insert(int x) {
int key1 = hash1(x), key2 = hash2(x);
for (int i = 0; i < max_loop; i++) {
if (h[0][key1].empty()) { // 如果第一个哈希表该位置为空,插入元素
h[0][key1].push_back(x);
return;
}
else if (h[0][key1][0] == x) // 如果元素已经存在,返回
return;
else {
int t = h[0][key1][0]; // 保存当前位置的元素
h[0][key1][0] = x; // 将元素插入哈希表
x = t; // 把原来的元素放到第二个哈希表中
key1 = hash2(t); // 更新哈希表位置
}
if (h[1][key2].empty()) { // 同上,只不过这里是第二个哈希表
h[1][key2].push_back(x);
return;
}
else if (h[1][key2][0] == x)
return;
else {
int t = h[1][key2][0];
h[1][key2][0] = x;
x = t;
key2 = hash1(t);
}
}
cout << "Insert Failed!" << endl; // 插入失败
}
// 查找元素
bool find(int x) {
int key1 = hash1(x), key2 = hash2(x);
for (int i = 0; i < max_loop; i++) {
if (h[0][key1].empty())
return false;
else if (h[0][key1][0] == x)
return true;
else {
key1 = hash2(h[0][key1][0]);
}
if (h[1][key2].empty())
return false;
else if (h[1][key2][0] == x)
return true;
else {
key2 = hash1(h[1][key2][0]);
}
}
return false;
}
// 删除元素
bool remove(int x) {
int key1 = hash1(x), key2 = hash2(x);
for (int i = 0; i < max_loop; i++) {
if (h[0][key1].empty())
return false;
else if (h[0][key1][0] == x) {
h[0][key1].pop_back();
return true;
}
else {
key1 = hash2(h[0][key1][0]);
}
if (h[1][key2].empty())
return false;
else if (h[1][key2][0] == x) {
h[1][key2].pop_back();
return true;
}
else {
key2 = hash1(h[1][key2][0]);
}
}
return false;
}
int main() {
cin >> n >> m;
int op, x;
for (int i = 1; i <= n; i++) {
cin >> x;
insert(x);
}
for (int i = 1; i <= m; i++) {
cin >> op >> x;
if (op == 1)
cout << (find(x) ? "Yes" : "No") << endl;
else
cout << (remove(x) ? "Success" : "Failed") << endl;
}
return 0;
}
```
其中,哈希函数的实现可以根据不同的应用场景进行调整。
### 回答2:
Cuckoo hash是一种哈希表的实现方式,它使用两个哈希函数和两个哈希表。下面是一个简单的Cuckoo hash代码示例。
```python
class CuckooHash:
def __init__(self, size):
self.size = size
self.table1 = [None] * size
self.table2 = [None] * size
def hash_function1(self, key):
return key % self.size
def hash_function2(self, key):
# 使用另一个哈希函数进行散列
return (key // self.size) % self.size
def insert(self, key):
index1 = self.hash_function1(key)
index2 = self.hash_function2(key)
if self.table1[index1] is None:
self.table1[index1] = key
elif self.table2[index2] is None:
self.table2[index2] = key
else:
# 需要重新哈希并重新插入
evicted_key = self.table1[index1]
self.table1[index1] = key
self.insert(evicted_key)
def search(self, key):
index1 = self.hash_function1(key)
index2 = self.hash_function2(key)
if self.table1[index1] == key:
return index1
elif self.table2[index2] == key:
return index2
else:
return -1
def remove(self, key):
index = self.search(key)
if index != -1:
if self.table1[index] == key:
self.table1[index] = None
else:
self.table2[index] = None
```
上述代码使用两个哈希表来存储数据,并通过两个哈希函数分别计算键的索引。在插入操作中,如果指定索引为空,则直接插入键。如果两个索引都已被占用,则需要从其中一个位置中踢出一个键,并使用另一个哈希函数将其重新插入。搜索操作会通过两个索引分别检查两个哈希表的位置。移除操作则是简单地将指定索引位置的键置为空。这样,Cuckoo hash能够更高效地解决哈希冲突问题。
### 回答3:
Cuckoo Hash是一种散列算法,用于解决散列表中的冲突问题。它的实现原理是使用两个散列函数以及两个散列表数组,分别表示两个哈希表。
首先,我们需要选择两个散列函数,这两个函数能够将输入的关键字映射到两个不同的散列表中。接下来,我们需要创建两个散列表数组,每个数组的大小都是固定的,可以根据实际应用进行选择。
在插入过程中,我们首先使用第一个散列函数将关键字映射到第一个散列表中,并将该关键字插入到对应的位置。如果该位置已经被占用,则我们需要将已存在的关键字重新映射到第二个散列表中,同时将新的关键字插入到第一个散列表中。这一过程可能会导致原本在第二个散列表中的关键字被挤出,并且需要将其重新插入到第一个散列表中。
类似地,我们使用第二个散列函数将挤出的关键字映射到第一个散列表中,并将其插入到对应的位置。如果该位置已经被占用,则需要将已存在的关键字重新映射到第二个散列表中,同时将新的关键字插入到第一个散列表中。这个过程会一直持续下去,直到找到一个未被占用的位置或者达到了最大插入次数的限制。
在查找过程中,我们使用两个散列函数分别在两个散列表中查找关键字。如果在其中一个散列表中找到了关键字,则表示该关键字存在。否则,我们可以认为该关键字不存在。
总的来说,Cuckoo Hash通过使用两个散列函数以及两个散列表数组,能够提供较低的查找时间复杂度,并且能够有效解决散列表中的冲突问题。它在一些特定的应用场景中能够提供更好的性能表现。
相关推荐
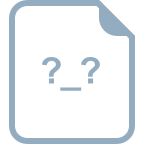














