写一个pytorch拟合一段非线性函数的程序
时间: 2023-08-31 18:19:38 浏览: 61
### 回答1:
好的,这是一个 PyTorch 程序例子,它拟合一段非线性函数:
```python
import torch
import matplotlib.pyplot as plt
# 定义非线性函数
def func(x):
return x**2 + 10*torch.sin(x)
# 生成训练数据
X = torch.unsqueeze(torch.linspace(-5, 5, 100), dim=1)
y = func(X)
# 定义模型
class Net(torch.nn.Module):
def __init__(self):
super(Net, self).__init__()
self.hidden = torch.nn.Linear(1, 20)
self.predict = torch.nn.Linear(20, 1)
def forward(self, x):
x = torch.relu(self.hidden(x))
x = self.predict(x)
return x
net = Net()
# 定义损失函数和优化器
criterion = torch.nn.MSELoss()
optimizer = torch.optim.SGD(net.parameters(), lr=0.2)
# 训练模型
for epoch in range(100):
prediction = net(X)
loss = criterion(prediction, y)
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 可视化结果
plt.figure()
plt.scatter(X.data.numpy(), y.data.numpy(), label='Original data')
plt.plot(X.data.numpy(), prediction.data.numpy(), label='Fitting Line')
plt.legend()
plt.show()
```
祝你好运!
### 回答2:
import torch
import torch.nn as nn
import torch.optim as optim
from torch.autograd import Variable
import numpy as np
import matplotlib.pyplot as plt
# 创建一个非线性函数
def nonlinear_function(x):
y = 0.5 * (x ** 2) + 2 * x + 1
return y
# 生成训练数据
x_train = torch.unsqueeze(torch.linspace(-5, 5, 100), dim=1)
y_train = nonlinear_function(x_train) + torch.randn(x_train.size()) * 0.1
# 定义神经网络模型
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.hidden = nn.Linear(1, 10)
self.relu = nn.ReLU()
self.output = nn.Linear(10, 1)
def forward(self, x):
x = self.hidden(x)
x = self.relu(x)
x = self.output(x)
return x
# 实例化模型
model = Net()
# 定义损失函数和优化器
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=0.01)
# 开始训练
num_epochs = 1000
for epoch in range(num_epochs):
inputs = Variable(x_train)
targets = Variable(y_train)
# 向前传播
output = model(inputs)
loss = criterion(output, targets)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (epoch+1) % 100 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
# 显示拟合结果
model.eval()
x_test = torch.unsqueeze(torch.linspace(-6, 6, 200), dim=1)
y_pred = model(Variable(x_test))
plt.plot(x_train.data.numpy(), y_train.data.numpy(), 'ro', label='Original data')
plt.plot(x_test.data.numpy(), y_pred.data.numpy(), 'b-', label='Fitted line')
plt.legend()
plt.show()
### 回答3:
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
# 定义非线性函数
def non_linear_func(x):
return torch.sin(x)
# 创建训练数据
x_train = np.random.uniform(-10, 10, size=(1000, 1))
y_train = non_linear_func(torch.Tensor(x_train)).numpy()
# 转换为Tensor
x_train = torch.Tensor(x_train)
y_train = torch.Tensor(y_train)
# 定义神经网络模型(单层全连接网络)
class NonLinearRegression(nn.Module):
def __init__(self):
super(NonLinearRegression, self).__init__()
self.fc = nn.Linear(1, 1) # 输入维度为1,输出维度为1
def forward(self, x):
x = self.fc(x)
return x
# 初始化模型和优化器
model = NonLinearRegression()
optimizer = optim.SGD(model.parameters(), lr=0.01)
loss_func = nn.MSELoss()
# 训练模型
for epoch in range(100):
y_pred = model(x_train)
loss = loss_func(y_pred, y_train)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (epoch+1) % 10 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, 100, loss.item()))
# 预测并可视化结果
import matplotlib.pyplot as plt
x_test = np.arange(-10, 10, 0.1)
x_test = torch.Tensor(x_test.reshape(-1, 1))
y_pred = model(x_test)
plt.scatter(x_train.numpy(), y_train.numpy(), color='b', label='Actual')
plt.plot(x_test.numpy(), y_pred.detach().numpy(), color='r', label='Predicted')
plt.legend()
plt.xlabel('x')
plt.ylabel('y')
plt.title('Nonlinear Regression')
plt.show()
相关推荐
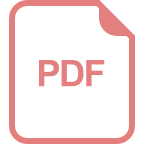
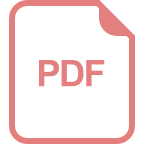
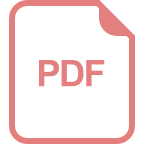














