使用Vue实例对象中的配置选项实现常用的案例效果。设置四个按钮,“话费”“机票”“酒店”“游戏” (2)每个按钮点击后对应文字“充话费”“订机票”“订酒店”“斗地主” (3)自定义盒子样式 (4)函数实现:点击按钮,自动切换盒子内的文字,同时切换按钮的颜色
时间: 2024-10-14 17:03:02 浏览: 23
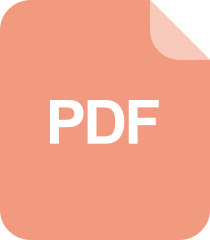
基于Vue实例对象的数据选项
在 Vue.js 中,你可以通过组件化的方式来创建这个需求。首先,创建一个名为 `Button` 的组件,并包含文本、颜色状态和点击事件处理。然后,在主应用组件 `App` 中使用这些按钮并绑定自定义的行为。
```html
<!-- Button.vue -->
<template>
<button :class="buttonStyle" @click="changeTextAndColor">
{{ buttonText }}
</button>
</template>
<script>
export default {
name: 'Button',
props: {
buttonText: String,
colorState: { // 颜色状态
type: String,
default: 'primary'
}
},
computed: {
buttonStyle() {
return {
backgroundColor: this.colorState,
padding: '10px 20px',
borderRadius: '5px'
};
}
},
methods: {
changeTextAndColor() {
// 根据索引切换文字和按钮颜色
const currentIndex = this.$index;
const newText = ['充话费', '订机票', '订酒店', '斗地主'][currentIndex];
this.buttonText = newText; // 更新文字
this.toggleColor(); // 自定义的切换颜色方法
},
toggleColor() {
// 示例:这里可以添加一个颜色数组来循环切换
const colors = ['primary', 'secondary', 'success', 'danger'];
const currentColor = this.colorState;
const newColorIndex = (colors.indexOf(currentColor) + 1) % colors.length;
this.colorState = colors[newColorIndex]; // 切换颜色
}
}
};
</script>
```
然后在 App.vue 或者你需要的地方引入并使用这个组件:
```html
<!-- App.vue -->
<template>
<div class="container">
<Button v-for="(item, index) in ['话费', '机票', '酒店', '游戏']" :key="index" :buttonText="item" />
</div>
</template>
<script>
import Button from './components/Button.vue';
export default {
components: {
Button
}
};
</script>
<style scoped>
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
</style>
```
在这个例子中,每次点击按钮,会触发 `changeTextAndColor` 方法,切换按钮的文字和颜色。你可以根据需要调整 `toggleColor` 方法来管理按钮的颜色变化规则。
阅读全文
相关推荐
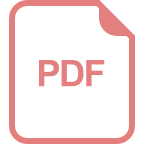
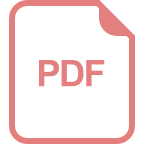
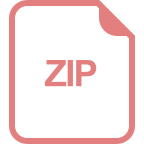
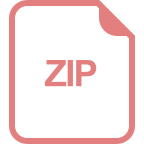
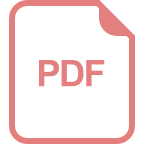
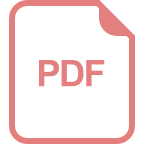
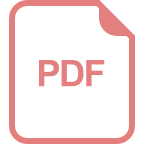
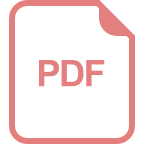
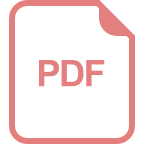
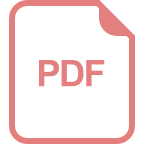
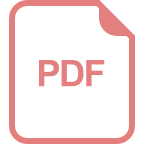
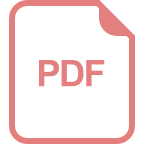
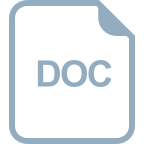
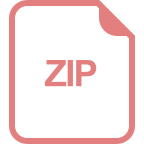
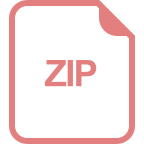
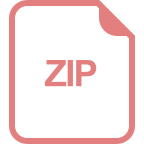
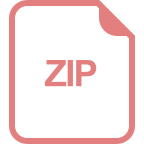