python tf.matmul 例子
时间: 2023-12-03 17:26:22 浏览: 29
好的,关于 python 的 tf.matmul 函数,您可以这样使用它:
import tensorflow as tf
a = tf.constant([[1, 2], [3, 4]])
b = tf.constant([[5, 6], [7, 8]])
c = tf.matmul(a, b)
with tf.Session() as sess:
result = sess.run(c)
print(result)
这个例子中,我们首先定义了两个矩阵 a 和 b,然后使用 tf.matmul 函数计算它们的乘积 c。最后,我们使用 TensorFlow 的 session 运行计算,并打印出结果。请注意,在 TensorFlow 中,所有的计算都是建立在计算图上,需要使用 session 来执行计算。
相关问题
tf.train.Saver
在 TensorFlow 中,`tf.train.Saver` 是用于保存和恢复模型参数的类。它可以将模型的变量保存到一个二进制的 checkpoint 文件中,也可以从 checkpoint 文件中恢复模型的变量。
`tf.train.Saver` 的用法如下:
```python
saver = tf.train.Saver(var_list=None, max_to_keep=5, keep_checkpoint_every_n_hours=10000.0, name=None, restore_sequentially=False, saver_def=None, builder=None, defer_build=False, allow_empty=False, sharded=False, write_version=tf.train.SaverDef.V2, pad_step_number=False, save_relative_paths=False, filename=None)
```
其中,`var_list` 参数指定需要保存或恢复的变量列表,如果不指定,则默认保存或恢复所有变量。`max_to_keep` 参数指定最多保存的 checkpoint 文件数量,`keep_checkpoint_every_n_hours` 参数指定保存 checkpoint 文件的时间间隔,`name` 参数指定 saver 的名称。
保存模型的变量:
```python
import tensorflow as tf
# 创建计算图
x = tf.placeholder(tf.float32, shape=[None, 784])
y = tf.placeholder(tf.float32, shape=[None, 10])
W = tf.Variable(tf.zeros([784, 10]))
b = tf.Variable(tf.zeros([10]))
logits = tf.matmul(x, W) + b
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y, logits=logits))
train_op = tf.train.GradientDescentOptimizer(0.5).minimize(loss)
# 训练模型
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for i in range(1000):
batch_xs, batch_ys = ...
sess.run(train_op, feed_dict={x: batch_xs, y: batch_ys})
# 保存模型参数
saver = tf.train.Saver()
saver.save(sess, './model.ckpt')
```
在这个例子中,我们创建了一个包含一个全连接层的简单神经网络,并使用梯度下降法训练模型。在训练完成后,我们调用 `tf.train.Saver` 类的 `save` 方法将模型的参数保存到文件 `'./model.ckpt'` 中。
恢复模型的变量:
```python
import tensorflow as tf
# 创建计算图
x = tf.placeholder(tf.float32, shape=[None, 784])
y = tf.placeholder(tf.float32, shape=[None, 10])
W = tf.Variable(tf.zeros([784, 10]))
b = tf.Variable(tf.zeros([10]))
logits = tf.matmul(x, W) + b
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y, logits=logits))
train_op = tf.train.GradientDescentOptimizer(0.5).minimize(loss)
# 恢复模型参数
saver = tf.train.Saver()
with tf.Session() as sess:
saver.restore(sess, './model.ckpt')
# 使用模型进行预测
test_x, test_y = ...
predictions = sess.run(logits, feed_dict={x: test_x})
```
在这个例子中,我们创建了与之前相同的计算图,并使用 `tf.train.Saver` 类的 `restore` 方法从文件 `'./model.ckpt'` 中恢复模型的参数。恢复参数后,我们可以使用模型进行预测。需要注意的是,恢复模型参数时,需要在调用 `tf.global_variables_initializer()` 之前调用 `saver.restore` 方法。
tf.keras.layers.Layer
tf.keras.layers.Layer是所有Keras层的基类,它继承自tf.Module。通过继承tf.keras.layers.Layer类,我们可以定义自己的层,并在模型中使用它们。在自定义层的类中,我们需要实现build方法和call方法。
build方法在构建模型时被调用,用于创建变量(权重)。该方法只会被调用一次,并在使用输入形状时调用。我们可以在build方法中定义并初始化我们需要的变量。
call方法定义了前向传播的计算。在该方法中,我们可以使用已定义的变量进行计算,并返回计算结果。
下面是一个使用tf.keras.layers.Layer的例子:
```python
import tensorflow as tf
class MyLayer(tf.keras.layers.Layer):
def __init__(self, output_dim, **kwargs):
self.output_dim = output_dim
super(MyLayer, self).__init__(**kwargs)
def build(self, input_shape):
self.w = tf.Variable(tf.random.normal([input_shape[-1], self.output_dim]), name='w')
self.b = tf.Variable(tf.zeros([self.output_dim]), name='b')
def call(self, inputs):
return tf.matmul(inputs, self.w) + self.b
# 实例化自定义层
my_layer = MyLayer(output_dim=3)
# 使用自定义层
x = tf.constant([[2.0,2.0, 2.0]])
output = my_layer(x)
print(output) # 输出计算结果
```
以上代码中,我们定义了一个名为MyLayer的自定义层,它有一个参数output_dim。在build方法中,我们创建了变量w和b,并在call方法中进行了计算。
相关推荐
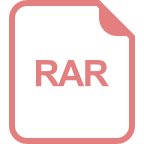












