用c语言编写一段代码 要求对单词采用递归下降分析方法进行语法分析,对不符合给定的文法的字符串,给出错误信息。
时间: 2024-02-29 11:52:44 浏览: 55
好的,以下是一个简单的使用C语言实现递归下降分析的示例代码,供您参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_LENGTH 100 // 定义单词最大长度
char lookahead; // 当前输入字符
char word[MAX_LENGTH]; // 当前单词
int index = 0; // 当前单词指针
// 判断当前字符是否为空格
int is_blank(char c) {
return c == ' ' || c == '\t' || c == '\n';
}
// 获取下一个字符
void get_char() {
lookahead = getchar();
}
// 获取下一个单词
void get_word() {
index = 0;
while (is_blank(lookahead)) {
get_char();
}
while (!is_blank(lookahead) && index < MAX_LENGTH - 1) {
word[index++] = lookahead;
get_char();
}
word[index] = '\0';
}
// 匹配当前单词,如果不匹配则输出错误信息
void match(char* expected) {
if (strcmp(word, expected) == 0) {
get_word();
} else {
printf("Error: expected %s but got %s\n", expected, word);
exit(1);
}
}
// 递归下降分析
void E();
void T();
void F();
void E() {
T();
while (strcmp(word, "+") == 0) {
match("+");
T();
}
}
void T() {
F();
while (strcmp(word, "*") == 0) {
match("*");
F();
}
}
void F() {
if (strcmp(word, "(") == 0) {
match("(");
E();
match(")");
} else {
match("id");
}
}
int main() {
printf("Please input your expression: ");
get_word();
E();
if (strcmp(word, "") != 0) {
printf("Error: unexpected symbol %s\n", word);
exit(1);
}
printf("Expression is correct!\n");
return 0;
}
```
这个示例程序实现了对简单的算术表达式的语法分析,包括加法和乘法运算。程序通过递归下降分析实现,每个非终结符对应一个函数,每个终结符对应一个match函数。程序读取输入的单词,并根据当前单词进行分析,如果不符合文法,则输出错误信息。
阅读全文
相关推荐


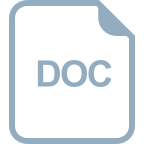
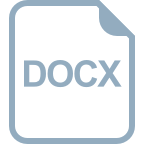
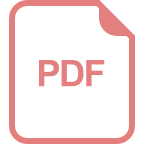
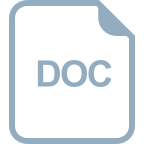
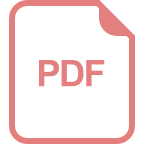
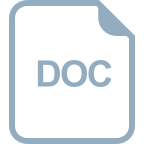
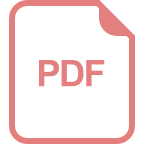
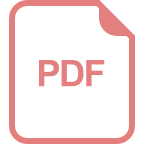
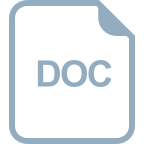
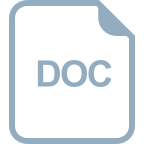
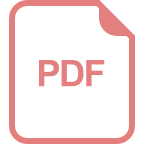
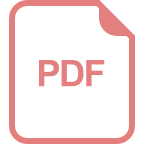
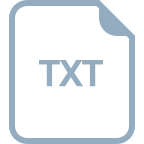
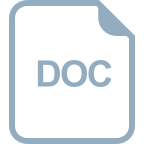