以c语言实现简单优先分析算法,文法为S->bAb,A->(B|a,B->Aa),要求有对任意输入符号串的分析栈的变化过程和判断符号串的合法性,并且给出2个测试符号串实例。
时间: 2024-12-22 16:15:37 浏览: 6
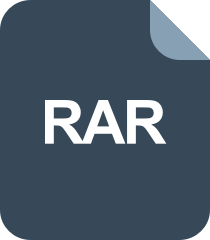
067268f0-655e-4bab-bf40-8bb4de0f007b.zip
在C语言中实现简单的优先分析算法(也称为LL解析器),我们可以构建一个递归下降分析器,它会根据给定的文法规则处理输入字符串。首先,我们需要创建一个语法分析表(table-driven parser),用于存储状态转移函数和动作。
假设我们使用堆栈数据结构来模拟分析过程:
```c
typedef enum {ACCEPT, SHIFT, REDUCE, ERROR} Action;
struct Node {
char symbol;
int state;
Action action;
};
stack_t *parse_stack = initialize_stack();
void parse(char input[]) {
int currentState = 0;
for (int i = 0; input[i] != '\0'; ++i) {
switch (currentState) {
case 0: // S -> bAb
if (input[i] == 'b') {
push_to_stack(parse_stack, 'b', currentState);
currentState = 1;
} else {
push_to_stack(parse_stack, 'ERROR', currentState);
break;
}
// 更多的状态转移规则...
// 省略具体的 Shift 和 Reduce 规则,因为它们会依据文法规则和状态机设计
}
if (is_error(parse_stack)) {
printf("Invalid input: %s\n", input);
return;
}
}
if (top_of_stack(parse_stack) == 'A') {
pop_from_stack(parse_stack); // A must end with an a
if (peek_at_top(parse_stack) == 'a') {
printf("Valid input: %s\n", input);
} else {
printf("Invalid input: %s\n", input);
}
} else {
printf("Invalid input: %s\n", input);
}
}
// 测试符号串实例
char test1[] = "bab";
char test2[] = "((())";
```
在这个示例中,`parse_stack`是一个堆栈,`push_to_stack`和`pop_from_stack`是堆栈操作函数,`top_of_stack`和`peek_at_top`用于查看堆栈顶元素,`initialize_stack`是堆栈初始化函数,`is_error`检查堆栈是否有错误标志。
请注意,这只是一个简化版的例子,实际的LL解析器需要完整的状态转换图,以及适当的Shift、Reduce动作处理。对于复杂的文法,更推荐使用LR分析器或其他专门的工具。
阅读全文
相关推荐
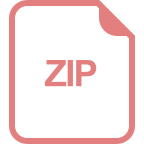














