用java编程序对于给定的文件sample.txt,统计其中单词的个数,以及给定单词出现的次数。
时间: 2023-11-27 15:50:07 浏览: 61
可以通过以下代码实现:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class WordCounter {
public static void main(String[] args) {
String filePath = "sample.txt";
String targetWord = "word"; // 要统计的单词
// 统计单词数量
int wordCount = countWords(filePath);
System.out.println("单词总数: " + wordCount);
// 统计目标单词出现次数
int targetWordCount = countTargetWord(filePath, targetWord);
System.out.println(targetWord + " 出现次数: " + targetWordCount);
}
/**
* 统计文件中单词的数量
* @param filePath 文件路径
* @return 单词数量
*/
public static int countWords(String filePath) {
int count = 0;
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
String[] words = line.split("\\s+");
count += words.length;
}
} catch (IOException e) {
e.printStackTrace();
}
return count;
}
/**
* 统计文件中指定单词的出现次数
* @param filePath 文件路径
* @param targetWord 目标单词
* @return 目标单词出现次数
*/
public static int countTargetWord(String filePath, String targetWord) {
int count = 0;
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
Map<String, Integer> wordMap = new HashMap<>();
String line;
while ((line = br.readLine()) != null) {
String[] words = line.split("\\s+");
for (String word : words) {
wordMap.put(word, wordMap.getOrDefault(word, 0) + 1);
}
}
count = wordMap.getOrDefault(targetWord, 0);
} catch (IOException e) {
e.printStackTrace();
}
return count;
}
}
```
其中,`countWords`方法用于统计文件中单词的数量,`countTargetWord`方法用于统计文件中指定单词的出现次数,两个方法都使用了`BufferedReader`来逐行读取文件内容,并通过`split`方法将每行文本按照空格分割成单词数组。在`countWords`方法中,我们只需要将每行单词数量累加即可;在`countTargetWord`方法中,我们使用了`Map`来统计每个单词出现的次数,并返回指定单词的出现次数。最后在`main`方法中调用这两个方法,并输出结果。
阅读全文
相关推荐
















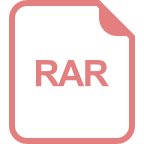