Java对于给定的文件sample.txt,统计其中单词的个数,以及给定单词出现的次数。
时间: 2023-11-27 16:50:00 浏览: 37
可以使用Java的文件读取和字符串操作来实现这个需求。具体步骤如下:
1. 使用Java的文件读取API读取sample.txt文件内容,并保存到一个字符串变量中。
2. 将读取到的字符串按照空格分割成单词,并保存到一个字符串数组中。
3. 统计字符串数组的长度,即为单词的个数。
4. 遍历字符串数组,统计每个单词出现的次数,并将结果保存到一个HashMap中。
下面是一个示例代码:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashMap;
public class WordCount {
public static void main(String[] args) {
String filename = "sample.txt";
String content = "";
try (BufferedReader br = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = br.readLine()) != null) {
content += line + " ";
}
} catch (IOException e) {
e.printStackTrace();
}
String[] words = content.split("\\s+");
System.out.println("单词个数:" + words.length);
HashMap<String, Integer> wordCount = new HashMap<>();
for (String word : words) {
if (wordCount.containsKey(word)) {
wordCount.put(word, wordCount.get(word) + 1);
} else {
wordCount.put(word, 1);
}
}
System.out.println("单词出现次数:");
for (String word : wordCount.keySet()) {
System.out.println(word + ": " + wordCount.get(word));
}
}
}
```
在该代码中,首先使用BufferedReader读取文件内容并保存到字符串变量content中。接着,使用split方法将content按照空格分割成字符串数组words,并统计其长度作为单词个数。
最后,使用HashMap统计每个单词出现的次数,并遍历输出结果。注意,在统计时需要判断该单词是否已经存在于HashMap中,如果存在则将其出现次数加1,否则将其出现次数设为1。
相关推荐
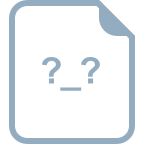
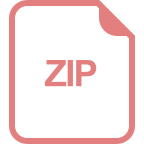














