编写c++程序定义一个矩形类,公有成员函数用于计算矩形的面积和周长。在主函数中输入矩形的宽和高,通过调用矩形类的成员函数来计算矩形的面积和周长。思考:如果类中成员变量矩形宽和矩形高定义为公有的或私有的,在编程时各自怎样实现。
时间: 2024-10-08 11:04:14 浏览: 57
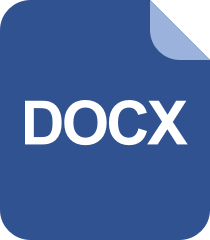
C++各套试题答案
在C++中,我们可以创建一个名为Rectangle的类来表示矩形,包含两个私有变量`width`和`height`,分别代表矩形的宽度和高度。为了保护数据安全并避免直接修改,我们将它们设置为私有,并提供公有成员函数`getArea()`和`perimeter()`来获取面积和周长。
```cpp
class Rectangle {
private:
double width;
double height;
public:
// 构造函数,初始化矩形的宽度和高度
Rectangle(double w, double h) : width(w), height(h) {}
// 公有函数,计算并返回矩形的面积
double getArea() const {
return width * height;
}
// 公有函数,计算并返回矩形的周长
double perimeter() const {
return 2 * (width + height);
}
};
int main() {
// 主函数中,获取用户输入的矩形尺寸
double inputWidth, inputHeight;
std::cout << "Enter the length of the rectangle: ";
std::cin >> inputWidth;
std::cout << "Enter the width of the rectangle: ";
std::cin >> inputHeight;
// 创建矩形对象并调用其成员函数
Rectangle rectangle(inputWidth, inputHeight);
double area = rectangle.getArea();
double perimeter = rectangle.perimeter();
std::cout << "The area of the rectangle is: " << area << "\n";
std::cout << "The perimeter of the rectangle is: " << perimeter << "\n";
return 0;
}
```
关于公有和私有属性的选择,如果我们需要外部访问和操作矩形的宽和高(如上述示例),则将它们设为公有。然而,如果想要保护数据不受意外修改,使其仅能通过特定的方式进行改变(比如只允许通过构造函数赋值),则可以将其设为私有并提供getter(如`getWidth()`和`getHeight()`)和setter(如`setWidth()`和`setHeight()`)方法。在这个例子中,我们选择了后者,因为我们的需求主要是通过已知的宽度和高度计算其他值,而不是让用户随意修改。
阅读全文
相关推荐
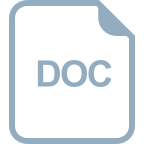
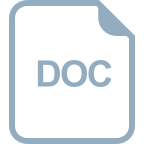
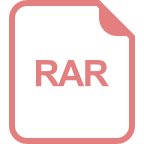
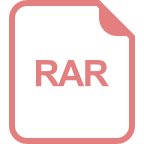













