用C++编写项目完整代码,设计三个图形类:Circle(圆)、Rectangle(矩形)、Triangle(三角形); 1、Cirlce 类基本信息:圆心坐标、半径; Rectangle 类基本信息:长、宽; Triangle 类基本信息:三个顶点坐标; 其中:成员变量为 private 属性,成员函数为public 属性; 2、每个图形类有多个构造函数:缺省构造函数、带参数的构造函数; 3、每个图形类有计算图形的面积GetArea(),显示图形的基本信息函数Show(),修改基本信息的函数Set(形参)。以Circle 类为例:通过GetArea()计算圆的面积,Show()函数中显示圆心坐标、直径、周长、面积等基本信息;Set(int x,int y, int r)函数可以修改圆心坐标和半径。 [实验提示] 1、注意多个构造函数的声明方式与定义实现; 2、当输入数据为不合理数据时(例如:输入的三角形的顶点是否能组成一个三角形), 提示用户输入错误; [测试数据] 1、Circle 类测试数据: 圆心:(20,30) 半径:5 (-20,40) 30 (40,-10) -5 2、Rectangle 类测试数据: 顶点坐标:20,50 40,5 -9,10 43,-8 3、Triangle 类测试数据: 顶点:(20,30)、(40,50)、(40,20) (10,10)、(50,10)、(35,10)
时间: 2024-03-22 14:42:14 浏览: 129
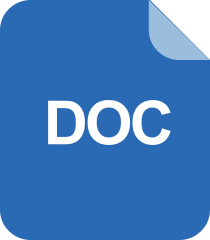
C++课程设计之三角形的种类和面积
抱歉,我无法提供完整的代码。但我可以给你一些思路:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Circle {
private:
int x, y; // 圆心坐标
int r; // 半径
public:
Circle() { // 缺省构造函数
x = y = r = 0;
}
Circle(int x, int y, int r) { // 带参数的构造函数
this->x = x;
this->y = y;
this->r = r;
}
double GetArea() { // 计算圆的面积
return M_PI * r * r;
}
void Show() { // 显示圆的基本信息
cout << "圆心坐标:(" << x << ", " << y << ")" << endl;
cout << "半径:" << r << endl;
cout << "直径:" << 2 * r << endl;
cout << "周长:" << 2 * M_PI * r << endl;
cout << "面积:" << GetArea() << endl;
}
void Set(int x, int y, int r) { // 修改圆的基本信息
this->x = x;
this->y = y;
this->r = r;
}
};
class Rectangle {
private:
int x1, y1; // 左上角顶点坐标
int x2, y2; // 右下角顶点坐标
public:
Rectangle() { // 缺省构造函数
x1 = y1 = x2 = y2 = 0;
}
Rectangle(int x1, int y1, int x2, int y2) { // 带参数的构造函数
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
}
double GetArea() { // 计算矩形的面积
return abs(x2 - x1) * abs(y2 - y1);
}
void Show() { // 显示矩形的基本信息
cout << "左上角顶点坐标:(" << x1 << ", " << y1 << ")" << endl;
cout << "右下角顶点坐标:(" << x2 << ", " << y2 << ")" << endl;
cout << "长:" << abs(x2 - x1) << endl;
cout << "宽:" << abs(y2 - y1) << endl;
cout << "面积:" << GetArea() << endl;
}
void Set(int x1, int y1, int x2, int y2) { // 修改矩形的基本信息
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
}
};
class Triangle {
private:
int x1, y1; // 第一个顶点坐标
int x2, y2; // 第二个顶点坐标
int x3, y3; // 第三个顶点坐标
public:
Triangle() { // 缺省构造函数
x1 = y1 = x2 = y2 = x3 = y3 = 0;
}
Triangle(int x1, int y1, int x2, int y2, int x3, int y3) { // 带参数的构造函数
// 判断三个顶点是否能组成一个三角形
if ((x1 - x2) * (y1 - y3) != (x1 - x3) * (y1 - y2)) {
cout << "输入的三角形顶点不合法!" << endl;
exit(0);
}
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
this->x3 = x3;
this->y3 = y3;
}
double GetArea() { // 计算三角形的面积
double a = sqrt(pow(x1 - x2, 2) + pow(y1 - y2, 2));
double b = sqrt(pow(x1 - x3, 2) + pow(y1 - y3, 2));
double c = sqrt(pow(x2 - x3, 2) + pow(y2 - y3, 2));
double p = (a + b + c) / 2;
return sqrt(p * (p - a) * (p - b) * (p - c));
}
void Show() { // 显示三角形的基本信息
cout << "第一个顶点坐标:(" << x1 << ", " << y1 << ")" << endl;
cout << "第二个顶点坐标:(" << x2 << ", " << y2 << ")" << endl;
cout << "第三个顶点坐标:(" << x3 << ", " << y3 << ")" << endl;
cout << "面积:" << GetArea() << endl;
}
void Set(int x1, int y1, int x2, int y2, int x3, int y3) { // 修改三角形的基本信息
// 判断三个顶点是否能组成一个三角形
if ((x1 - x2) * (y1 - y3) != (x1 - x3) * (y1 - y2)) {
cout << "输入的三角形顶点不合法!" << endl;
exit(0);
}
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
this->x3 = x3;
this->y3 = y3;
}
};
int main() {
Circle c1(20, 30, 5);
c1.Show();
c1.Set(-20, 40, 30);
c1.Show();
Rectangle r1(20, 50, 40, 5);
r1.Show();
r1.Set(-9, 10, 43, -8);
r1.Show();
Triangle t1(20, 30, 40, 50, 40, 20);
t1.Show();
t1.Set(10, 10, 50, 10, 35, 10);
t1.Show();
return 0;
}
```
这是一个简单的面向对象的实现,包括三个类:Circle、Rectangle、Triangle,每个类中都有三个私有属性和公有函数(构造函数、GetArea()、Show()、Set(形参)),还有一个 main 函数用于测试类的功能。
注意:在 Triangle 类中,判断三个顶点是否能组成一个三角形的公式为:(x1 - x2) * (y1 - y3) != (x1 - x3) * (y1 - y2)。如果成立,则可以组成一个三角形;否则不行。
阅读全文
相关推荐
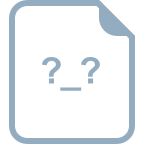










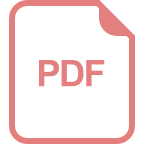
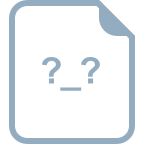