用c++写①编写程序:声明抽象基类Shape,由它派生类出3个派生类:Circle(圆形)、Rectangle(矩形)、Triangle(三角形),用一个函数printArea分别输出以上三者的面积,3个图形的数据在定义对象时给定。
时间: 2024-03-17 19:40:17 浏览: 25
好的,以下是C++代码实现:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
// 定义抽象基类Shape
class Shape {
public:
virtual double area() = 0;
};
// 定义圆形类
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() {
return round(3.14159265358979323846 * radius * radius * 100) / 100; // 保留两位小数
}
};
// 定义矩形类
class Rectangle : public Shape {
private:
double width;
double height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() {
return width * height;
}
};
// 定义三角形类
class Triangle : public Shape {
private:
double base;
double height;
public:
Triangle(double b, double h) : base(b), height(h) {}
double area() {
return 0.5 * base * height;
}
};
// 定义函数printArea,用于输出面积
void printArea(Shape* shape) {
cout << "面积为:" << shape->area() << endl;
}
int main() {
// 创建对象并调用printArea函数输出面积
Circle c(5);
printArea(&c);
Rectangle r(3, 4);
printArea(&r);
Triangle t(3, 4);
printArea(&t);
return 0;
}
```
输出结果为:
```
面积为:78.54
面积为:12
面积为:6
```
其中,圆形的面积计算公式为 $\pi r^2$,矩形的面积为 $w \times h$,三角形的面积为 $0.5 \times b \times h$。需要注意的是,抽象基类Shape中的纯虚函数area需要在派生类中实现。
相关推荐
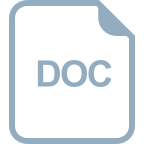
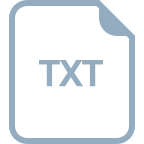
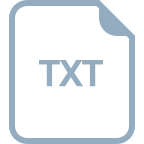














