C++设计三个图形类:Circle(圆)、Rectangle(矩形)、Triangle(三角形); 1、Cirlce 类基本信息:圆心坐标、半径; Rectangle 类基本信息:长、宽; Triangle 类基本信息:三个顶点坐标; 其中:成员变量为 private 属性,成员函数为public 属性; 2、每个图形类有多个构造函数:缺省构造函数、带参数的构造函数; 3、每个图形类有计算图形的面积GetArea(),显示图形的基本信息函数Show(),修改基本信息的函数Set(形参)。以Circle 类为例:通过GetArea()计算圆的面积,Show()函数中显示圆心坐标、直径、周长、面积等基本信息;Set(int x,int y, int r)函数可以修改圆心坐标和半径。
时间: 2024-02-18 09:05:15 浏览: 27
好的,我可以为您解答这个问题。
首先,我们需要定义三个图形类:Circle、Rectangle、Triangle。
```c++
class Circle {
private:
int x; // 圆心 x 坐标
int y; // 圆心 y 坐标
int r; // 半径
public:
Circle();
Circle(int x, int y, int r);
double GetArea();
void Show();
void Set(int x, int y, int r);
};
class Rectangle {
private:
int length; // 长
int width; // 宽
public:
Rectangle();
Rectangle(int length, int width);
double GetArea();
void Show();
void Set(int length, int width);
};
class Triangle {
private:
int x1, y1; // 第一个顶点坐标
int x2, y2; // 第二个顶点坐标
int x3, y3; // 第三个顶点坐标
public:
Triangle();
Triangle(int x1, int y1, int x2, int y2, int x3, int y3);
double GetArea();
void Show();
void Set(int x1, int y1, int x2, int y2, int x3, int y3);
};
```
然后,我们需要实现每个类的构造函数、GetArea()、Show()和Set()函数。
Circle 类的实现如下:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const double PI = 3.141592653589793;
Circle::Circle() {
x = 0;
y = 0;
r = 0;
}
Circle::Circle(int x, int y, int r) {
this->x = x;
this->y = y;
this->r = r;
}
double Circle::GetArea() {
return PI * r * r;
}
void Circle::Show() {
cout << "圆心坐标:(" << x << ", " << y << ")" << endl;
cout << "直径: " << 2 * r << endl;
cout << "周长: " << 2 * PI * r << endl;
cout << "面积: " << GetArea() << endl;
}
void Circle::Set(int x, int y, int r) {
this->x = x;
this->y = y;
this->r = r;
}
```
Rectangle 类的实现如下:
```c++
#include <iostream>
using namespace std;
Rectangle::Rectangle() {
length = 0;
width = 0;
}
Rectangle::Rectangle(int length, int width) {
this->length = length;
this->width = width;
}
double Rectangle::GetArea() {
return length * width;
}
void Rectangle::Show() {
cout << "长: " << length << endl;
cout << "宽: " << width << endl;
cout << "面积: " << GetArea() << endl;
}
void Rectangle::Set(int length, int width) {
this->length = length;
this->width = width;
}
```
Triangle 类的实现如下:
```c++
#include <iostream>
#include <cmath>
using namespace std;
Triangle::Triangle() {
x1 = 0;
y1 = 0;
x2 = 0;
y2 = 0;
x3 = 0;
y3 = 0;
}
Triangle::Triangle(int x1, int y1, int x2, int y2, int x3, int y3) {
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
this->x3 = x3;
this->y3 = y3;
}
double Triangle::GetArea() {
double a = sqrt(pow(x1 - x2, 2) + pow(y1 - y2, 2));
double b = sqrt(pow(x1 - x3, 2) + pow(y1 - y3, 2));
double c = sqrt(pow(x2 - x3, 2) + pow(y2 - y3, 2));
double p = (a + b + c) / 2;
return sqrt(p * (p - a) * (p - b) * (p - c));
}
void Triangle::Show() {
cout << "第一个顶点坐标:(" << x1 << ", " << y1 << ")" << endl;
cout << "第二个顶点坐标:(" << x2 << ", " << y2 << ")" << endl;
cout << "第三个顶点坐标:(" << x3 << ", " << y3 << ")" << endl;
cout << "面积: " << GetArea() << endl;
}
void Triangle::Set(int x1, int y1, int x2, int y2, int x3, int y3) {
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
this->x3 = x3;
this->y3 = y3;
}
```
这样,我们就完成了三个图形类的设计。可以通过调用相应的构造函数、GetArea()、Show()和Set()函数,来创建、计算、显示和修改相应的图形对象。
相关推荐
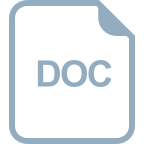
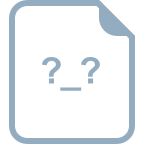













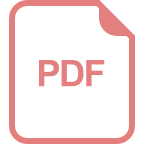