用C++设计一个图形类(Shape),由它派生出5个派生类:三角形类(Triangle)、正方形类(Square)、圆形类(Circle)、矩形类(Rectangle)、梯形类(triangle)类,利用虚函数计算图形面积,用一个函数printArea分别输出以上5者的面积
时间: 2024-01-11 17:02:02 浏览: 164
好的,以下是代码实现:
```c++
#include <iostream>
using namespace std;
class Shape {
public:
virtual double getArea() = 0;
};
class Triangle : public Shape {
public:
Triangle(double b, double h) : base(b), height(h) {}
double getArea() override {
return base * height / 2;
}
private:
double base;
double height;
};
class Square : public Shape {
public:
Square(double s) : side(s) {}
double getArea() override {
return side * side;
}
private:
double side;
};
class Circle : public Shape {
public:
Circle(double r) : radius(r) {}
double getArea() override {
return 3.1415 * radius * radius;
}
private:
double radius;
};
class Rectangle : public Shape {
public:
Rectangle(double w, double h) : width(w), height(h) {}
double getArea() override {
return width * height;
}
private:
double width;
double height;
};
class Trapezoid : public Shape {
public:
Trapezoid(double a, double b, double h) : upperBase(a), lowerBase(b), height(h) {}
double getArea() override {
return (upperBase + lowerBase) * height / 2;
}
private:
double upperBase;
double lowerBase;
double height;
};
void printArea(Shape* s) {
cout << "Area: " << s->getArea() << endl;
}
int main() {
Shape* s1 = new Triangle(3, 4);
printArea(s1);
Shape* s2 = new Square(5);
printArea(s2);
Shape* s3 = new Circle(3);
printArea(s3);
Shape* s4 = new Rectangle(4, 5);
printArea(s4);
Shape* s5 = new Trapezoid(1, 3, 2);
printArea(s5);
return 0;
}
```
这段代码中,我们首先定义了一个抽象类 Shape,它包含了一个纯虚函数 getArea(),用于计算图形的面积。然后我们分别定义了五个派生类 Triangle、Square、Circle、Rectangle 和 Trapezoid,它们分别实现了 Shape 类的 getArea() 函数,用于计算各自的面积。最后,我们定义了一个函数 printArea,用于输出各图形的面积,它接受一个指向 Shape 类型的指针作为参数,可以根据不同的派生类自动调用对应的 getArea() 函数。
在 main 函数中,我们分别创建了五个不同的对象,并调用了 printArea 函数输出它们的面积。由于 Shape 类型的指针可以指向任意一个派生类对象,因此我们可以通过一个函数完成多个派生类对象的输出,实现了代码的重用。
阅读全文
相关推荐
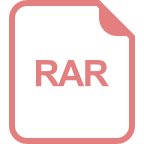
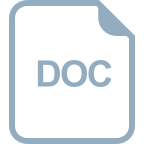















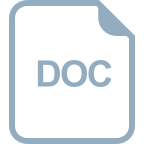