用C++实现以下功能。14. 定义复数类COMPLEX,并用成员函数为复数类COMPLEX重载加、减及赋值运算符操作。 15. 写一个程序,定义一个抽象类Shape,由它派生3个类:Square(正方形)、Trapezoid(梯形)和Triangle三角形。用虚函数分别计算几种图形面积、并求它们的和。要求用基类指针数组,使它每一个元素指向一个派生类对象。
时间: 2024-03-10 09:43:19 浏览: 21
14. COMPLEX类定义如下:
```c++
class COMPLEX {
private:
double real;
double imag;
public:
COMPLEX(double r = 0, double i = 0) : real(r), imag(i) {}
COMPLEX operator+(const COMPLEX& c) const {
return COMPLEX(real + c.real, imag + c.imag);
}
COMPLEX operator-(const COMPLEX& c) const {
return COMPLEX(real - c.real, imag - c.imag);
}
COMPLEX& operator=(const COMPLEX& c) {
if (this != &c) {
real = c.real;
imag = c.imag;
}
return *this;
}
};
```
15. Shape类定义如下:
```c++
class Shape {
public:
virtual double area() const = 0;
virtual ~Shape() {}
};
class Square : public Shape {
private:
double side;
public:
Square(double s = 0) : side(s) {}
double area() const {
return side * side;
}
};
class Trapezoid : public Shape {
private:
double upper;
double lower;
double height;
public:
Trapezoid(double u = 0, double l = 0, double h = 0) :
upper(u), lower(l), height(h) {}
double area() const {
return (upper + lower) * height / 2;
}
};
class Triangle : public Shape {
private:
double base;
double height;
public:
Triangle(double b = 0, double h = 0) : base(b), height(h) {}
double area() const {
return base * height / 2;
}
};
```
然后可以定义一个Shape类型的指针数组,每个元素指向一个派生类对象,计算它们的面积和,代码如下:
```c++
int main() {
Shape* shapes[3];
shapes[0] = new Square(5);
shapes[1] = new Trapezoid(3, 5, 4);
shapes[2] = new Triangle(4, 3);
double sum = 0;
for (int i = 0; i < 3; i++) {
sum += shapes[i]->area();
}
std::cout << "The sum of areas is: " << sum << std::endl;
for (int i = 0; i < 3; i++) {
delete shapes[i];
}
return 0;
}
```
相关推荐
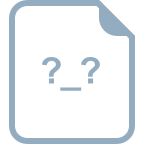
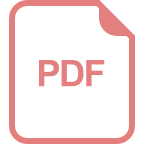














