用kotlin自定义一个显示商品信息的视图
时间: 2024-10-14 09:06:38 浏览: 24
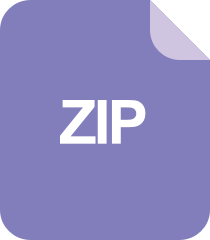
【路径规划】乌燕鸥算法栅格地图机器人最短路径规划【含Matlab仿真 2886期】.zip
在Kotlin中自定义一个显示商品信息的视图通常涉及到数据绑定和界面布局的设计。首先,你需要创建一个代表商品信息的数据类(Product),例如:
```kotlin
data class Product(
val name: String,
val price: Double,
val description: String
)
```
然后,你可以设计一个布局文件(通常是XML),比如`activity_product_detail.xml`,用于展示商品详情:
```xml
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<data>
<!-- 定义属性 -->
<variable
name="product"
type="Product" />
</data>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="@{product.name}"
android:textSize="20sp" />
<TextView
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="价格: $@{product.price}"
android:textSize="20sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@{product.description}"
android:textSize="16sp" />
</LinearLayout>
</layout>
```
在这个布局中,我们使用了Kotlin的数据绑定技术(`@{}`注解),它会将`Product`对象的数据自动映射到相应的视图上。
最后,在对应的Activity或Fragment中,通过`ViewModel`或`LiveData`来绑定并设置商品数据:
```kotlin
class ProductDetailViewModel : ViewModel() {
// 假设有一个LiveData来获取商品数据
private val productData = MutableLiveData<Product>()
fun showProduct(product: Product) {
productData.value = product
}
}
// 在Activity或Fragment中
val viewModel = ViewModelProvider(this).get(ProductDetailViewModel::class.java)
viewModel.showProduct(YourProductInstance)
// 在onCreateView或onBind中设置数据绑定
binding.productName.text = viewModel.productData.value?.name
binding.productPrice.text = "价格: $" + viewModel.productData.value?.price
// 等等...
```
阅读全文
相关推荐
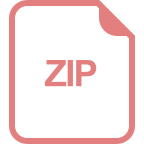
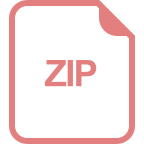
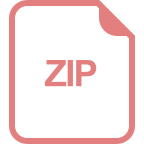
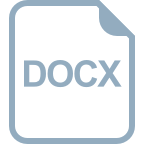
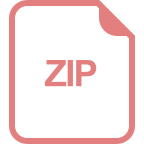
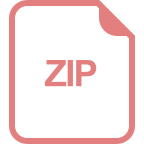
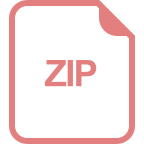
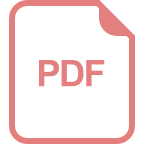
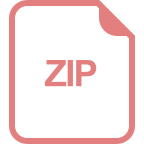
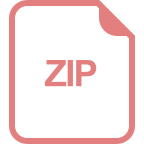
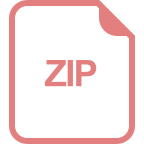
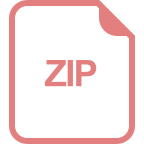
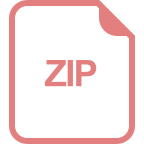
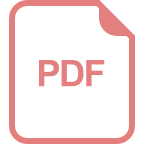
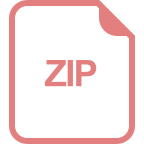
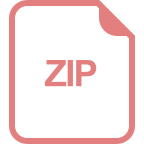
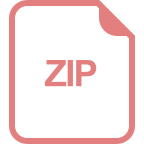